JavaScript: Break an address of an url and put it's part into an array
JavaScript Basic: Exercise-144 with Solution
Break URL into Parts
Write a JavaScript program to break an URL address and put its parts into an array.
Note: url structure : ://.org[/] and there may be no part in the address.
Visual Presentation:
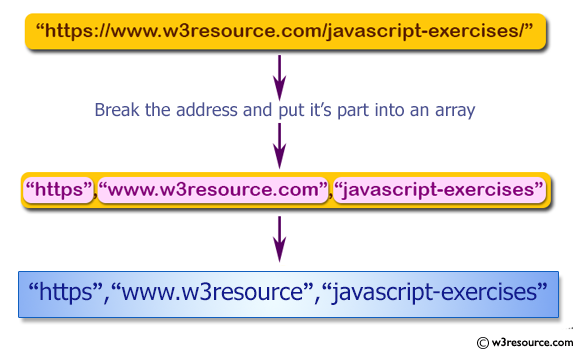
Sample Solution:
JavaScript Code:
/**
* Function to break down a URL address into protocol, domain, and optional path
* @param {string} url_add - The URL address to be broken down
* @returns {array} - An array containing protocol, domain, and optional path (if available)
*/
function break_address(url_add) {
// Splitting the URL to separate protocol and the rest of the address
var data = url_add.split("://");
var protocol = data[0];
// Splitting the rest of the address to extract domain and possible path
data = data[1].split(".com");
var domain = data[0];
// Splitting the address after .com to extract the path if available
data = data[1].split("/");
// Checking if a path exists and returning the relevant parts
if (data[1]) {
return [protocol, domain, data[1]];
}
// Returning protocol and domain if path doesn't exist
return [protocol, domain];
}
// Sample URL address
var url_add = "https://www.w3resource.com/javascript-exercises/";
// Display original address
console.log("Original address: " + url_add);
// Display the broken down parts of the address
console.log(break_address(url_add));
Output:
Original address: https://www.w3resource.com/javascript-exercises/ ["https","www.w3resource","javascript-exercises"]
Live Demo:
See the Pen javascript-basic-exercise-144 by w3resource (@w3resource) on CodePen.
Flowchart:
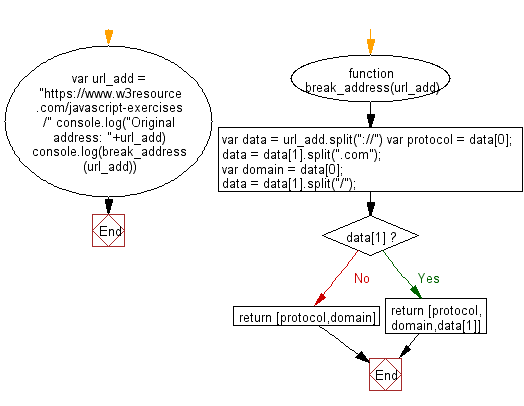
ES6 Version:
/**
* Function to break down a URL address into protocol, domain, and optional path
* @param {string} url_add - The URL address to be broken down
* @returns {array} - An array containing protocol, domain, and optional path (if available)
*/
const break_address = (url_add) => {
// Splitting the URL to separate protocol and the rest of the address
let data = url_add.split("://");
let protocol = data[0];
// Splitting the rest of the address to extract domain and possible path
data = data[1].split(".com");
let domain = data[0];
// Splitting the address after .com to extract the path if available
data = data[1].split("/");
// Checking if a path exists and returning the relevant parts
if (data[1]) {
return [protocol, domain, data[1]];
}
// Returning protocol and domain if path doesn't exist
return [protocol, domain];
};
// Sample URL address
const url_add = "https://www.w3resource.com/javascript-exercises/";
// Display original address
console.log("Original address: " + url_add);
// Display the broken down parts of the address
console.log(break_address(url_add));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to sort the strings of a given array of strings in the order of increasing lengths.
Next: JavaScript program to find the maximum integer n such that 1 + 2 + ... + n <= a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics