JavaScript: Simplify a given absolute path for a file in Unix-style
JavaScript Basic: Exercise-142 with Solution
Simplify Unix-Style Absolute File Path
Write a JavaScript program to simplify a given absolute path for a file in Unix-style.
Sample Solution:
JavaScript Code:
/**
* Function to simplify a given path
* @param {string} main_path - The main path to simplify
* @returns {string} - Returns the simplified path
*/
function simplify_path(main_path) {
// Splitting the path into parts
var parts = main_path.split('/'),
new_path = [], // Array to store the simplified path
length = 0; // Variable to keep track of the length of the new path
// Loop through each part of the path
for (var i = 0; i < parts.length; i++) {
var part = parts[i];
// Conditions to handle special cases ('.', '..', '')
if (part === '.' || part === '' || part === '..') {
if (part === '..' && length > 0) {
length--; // Move back one directory level
}
continue; // Skip to the next part
}
new_path[length++] = part; // Store the valid part in the new path array
}
// If the new path is empty, return the root path '/'
if (length === 0) {
return '/';
}
// Reconstructing the simplified path
var result = '';
for (var i = 0; i < length; i++) {
result += '/' + new_path[i]; // Append parts of the new path separated by '/'
}
return result; // Return the simplified path
}
console.log(simplify_path("/home/var/./www/../html//sql/")); // Output: '/home/var/html/sql'
Output:
/home/var/html/sql
Live Demo:
See the Pen javascript-basic-exercise-142 by w3resource (@w3resource) on CodePen.
Flowchart:
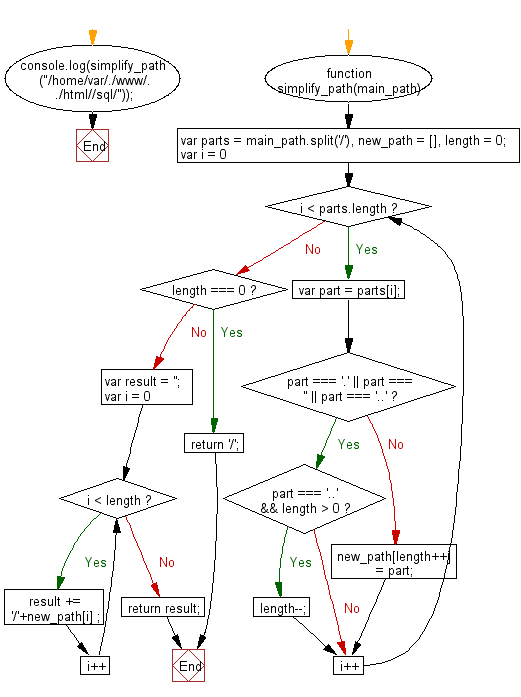
ES6 Version:
/**
* Function to simplify a given path
* @param {string} mainPath - The main path to simplify
* @returns {string} - Returns the simplified path
*/
const simplifyPath = (mainPath) => {
const parts = mainPath.split('/'); // Splitting the path into parts
const newPath = []; // Array to store the simplified path
let newPathLength = 0; // Variable to keep track of the length of the new path
// Loop through each part of the path
for (let i = 0; i < parts.length; i++) {
const part = parts[i];
// Conditions to handle special cases ('.', '..', '')
if (part === '.' || part === '' || part === '..') {
// Move back one directory level if '..' and there's a directory to move back to
if (part === '..' && newPathLength > 0) {
newPathLength--;
}
continue; // Skip to the next part
}
newPath[newPathLength++] = part; // Store the valid part in the new path array
}
// If the new path is empty, return the root path '/'
if (newPathLength === 0) {
return '/';
}
// Reconstructing the simplified path
let result = '';
for (let i = 0; i < newPathLength; i++) {
result += '/' + newPath[i]; // Append parts of the new path separated by '/'
}
return result; // Return the simplified path
}
console.log(simplifyPath("/home/var/./www/../html//sql/")); // Output: '/home/var/html/sql'
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the number of elements which presents in both of the given arrays.
Next: JavaScript program to sort the strings of a given array of strings in the order of increasing lengths.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics