JavaScript: Find the number of elements which presents in both of the given arrays
JavaScript Basic: Exercise-141 with Solution
Find Number of Elements in Both Arrays
Write a JavaScript program to find the number of elements in both arrays.
Visual Presentation:
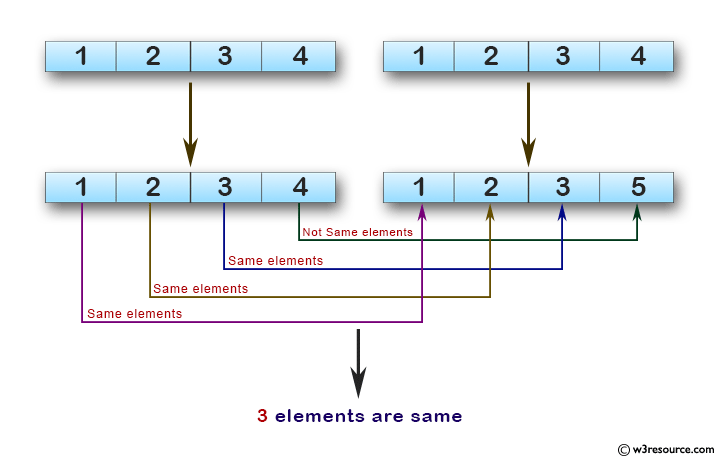
Sample Solution:
JavaScript Code:
/**
* Function to count the number of same elements in both arrays
* @param {Array} arra1 - The first input array
* @param {Array} arra2 - The second input array
* @returns {number} - Returns the count of same elements between the arrays
*/
function test_same_elements_both_arrays(arra1, arra2) {
var result = 0; // Counter to store the count of same elements
// Loop through the first array
for (var i = 0; i < arra1.length; i++) {
// Loop through the second array
for (var j = 0; j < arra2.length; j++) {
if (arra1[i] === arra2[j]) {
result++; // Increment the counter if the elements match
}
}
}
return result; // Return the count of same elements
}
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [1, 2, 3, 4])); // Output: 4
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [1, 2, 3, 5])); // Output: 3
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [11, 22, 33, 44])); // Output: 0
Output:
4 3 0
Live Demo:
See the Pen javascript-basic-exercise-141 by w3resource (@w3resource) on CodePen.
Flowchart:
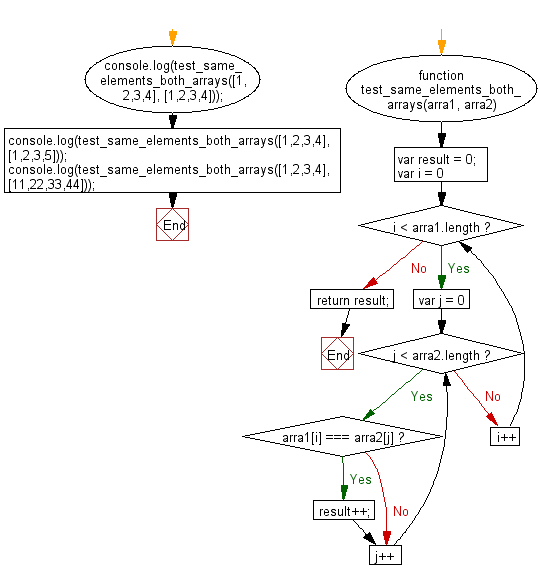
ES6 Version:
/**
* Function to count the number of same elements in both arrays
* @param {Array} arra1 - The first input array
* @param {Array} arra2 - The second input array
* @returns {number} - Returns the count of same elements between the arrays
*/
const test_same_elements_both_arrays = (arra1, arra2) => {
let result = 0; // Counter to store the count of same elements
// Loop through the first array
for (let i = 0; i < arra1.length; i++) {
// Loop through the second array
for (let j = 0; j < arra2.length; j++) {
if (arra1[i] === arra2[j]) {
result++; // Increment the counter if the elements match
}
}
}
return result; // Return the count of same elements
};
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [1, 2, 3, 4])); // Output: 4
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [1, 2, 3, 5])); // Output: 3
console.log(test_same_elements_both_arrays([1, 2, 3, 4], [11, 22, 33, 44])); // Output: 0
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the number of common elements shared between two arrays using set intersection.
- Write a JavaScript function that compares two arrays and returns the count of elements that appear in both.
- Write a JavaScript program that finds the intersection of two arrays and outputs the number of shared elements, accounting for duplicates.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if all the digits in a given number are the same or not.
Next: Simplify a given absolute path for a file in Unix-style.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.