JavaScript: Check whether all the digits in a given number are the same or not
JavaScript Basic: Exercise-140 with Solution
Check if All Digits in Number Are Same
Write a JavaScript program to check whether all the digits in a given number are the same or not.
Visual Presentation:
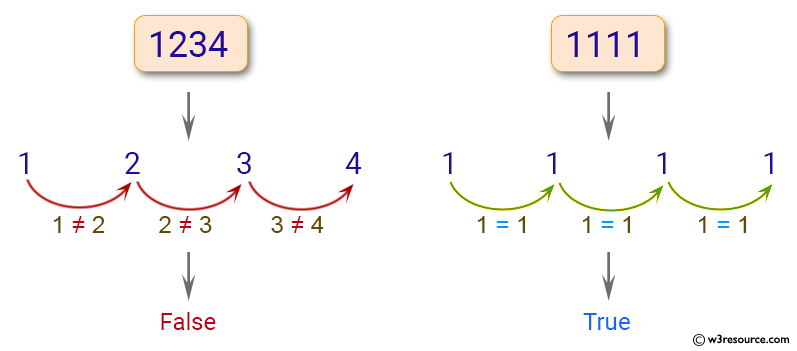
Sample Solution:
JavaScript Code:
/**
* Function to test if all digits in the number are the same
* @param {number} num - The input number
* @returns {boolean} - Returns true if all digits are the same, false otherwise
*/
function test_same_digit(num) {
var first = num % 10; // Extracting the last digit as a reference
while (num) {
if (num % 10 !== first) return false; // Checking if the current digit is different from the reference
num = Math.floor(num / 10); // Removing the last digit by integer division
}
return true; // Returning true if all digits are the same
}
console.log(test_same_digit(1234)); // Output: false
console.log(test_same_digit(1111)); // Output: true
console.log(test_same_digit(22222222)); // Output: true
Output:
false true true
Live Demo:
See the Pen javascript-basic-exercise-140 by w3resource (@w3resource) on CodePen.
Flowchart:
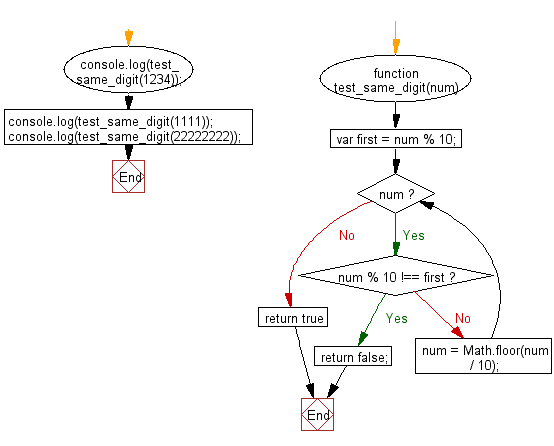
ES6 Version:
/**
* Function to test if all digits in the number are the same
* @param {number} num - The input number
* @returns {boolean} - Returns true if all digits are the same, false otherwise
*/
const test_same_digit = (num) => {
let first = num % 10; // Extracting the last digit as a reference
while (num) {
if (num % 10 !== first) return false; // Checking if the current digit is different from the reference
num = Math.floor(num / 10); // Removing the last digit by integer division
}
return true; // Returning true if all digits are the same
};
console.log(test_same_digit(1234)); // Output: false
console.log(test_same_digit(1111)); // Output: true
console.log(test_same_digit(22222222)); // Output: true
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines whether all digits in an integer are identical by comparing each digit.
- Write a JavaScript function that converts a number to a string and checks if every character matches the first digit.
- Write a JavaScript program that iterates over the digits of a number and returns true if they are all the same, false otherwise.
Go to:
PREV : Find Position of Rightmost Round Number.
NEXT : Find Number of Elements in Both Arrays.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.