JavaScript: Find the position of a rightmost round number in an array of integers. Returns 0 if there are no round number
JavaScript Basic: Exercise-139 with Solution
Find Position of Rightmost Round Number
Write a JavaScript program to find the position of the rightmost round number in an array of integers. If there are no round numbers, the function returns 0.
Note: A round number is informally considered to be an integer that ends with one or more zeros.
Visual Presentation:
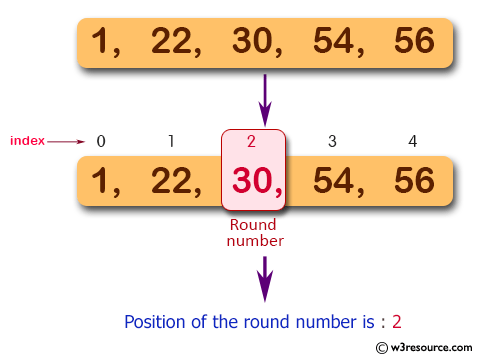
Sample Solution:
JavaScript Code:
/**
* Function to find the index of the rightmost round number (divisible by 10) in the array
* @param {number[]} input_arr - The input array of numbers
* @returns {number} - The index of the rightmost round number (if found)
*/
function find_rightmost_round_number(input_arr) {
var result = 0; // Variable to store the index of the rightmost round number (default: 0)
for (var i = 0; i < input_arr.length; i++) {
if (input_arr[i] % 10 === 0) { // Checking if the number is divisible by 10
result = i; // Updating 'result' with the current index if the number is divisible by 10
}
}
return result; // Returning the index of the rightmost round number
}
console.log(find_rightmost_round_number([1, 22, 30, 54, 56]));
console.log(find_rightmost_round_number([1, 22, 32, 54, 56]));
console.log(find_rightmost_round_number([1, 22, 32, 54, 50]));
Output:
2 0 4
Live Demo:
See the Pen javascript-basic-exercise-139 by w3resource (@w3resource) on CodePen.
Flowchart:
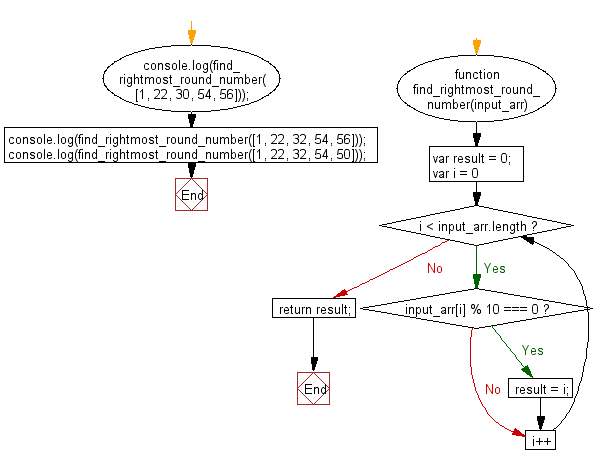
ES6 Version:
/**
* Function to find the index of the rightmost round number (divisible by 10) in the array
* @param {number[]} input_arr - The input array of numbers
* @returns {number} - The index of the rightmost round number (if found)
*/
const find_rightmost_round_number = (input_arr) => {
let result = 0; // Variable to store the index of the rightmost round number (default: 0)
for (let i = 0; i < input_arr.length; i++) {
if (input_arr[i] % 10 === 0) { // Checking if the number is divisible by 10
result = i; // Updating 'result' with the current index if the number is divisible by 10
}
}
return result; // Returning the index of the rightmost round number
}
console.log(find_rightmost_round_number([1, 22, 30, 54, 56]));
console.log(find_rightmost_round_number([1, 22, 32, 54, 56]));
console.log(find_rightmost_round_number([1, 22, 32, 54, 50]));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the index of the rightmost round number in an array (a number ending in zero).
- Write a JavaScript function that iterates through an array from the end and returns the position of the first element ending in zero.
- Write a JavaScript program that scans an array for round numbers and outputs the index of the last occurrence, or returns 0 if none are found.
Go to:
PREV : Reverse Bits in 16-Bit Unsigned Integer.
NEXT : Check if All Digits in Number Are Same.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.