JavaScript: Reverse the bits of a given 16 bits unsigned short integer
JavaScript Basic: Exercise-138 with Solution
Reverse Bits in 16-Bit Unsigned Integer
Write a JavaScript program to reverse the bits of a given 16-bit unsigned short integer.
Sample Solution:
JavaScript Code:
/**
* Function to reverse the binary representation of a 16-bit number
* @param {number} num - The input number
* @returns {number} - The reversed binary number
*/
function sixteen_bits_reverse(num) {
var result = 0; // Initialize result to store the reversed number
for (var i = 0; i < 16; i++) {
result = result * 2 + (num % 2); // Reversing the bits by shifting result left and adding the last bit of 'num'
num = Math.floor(num / 2); // Right-shifting 'num' to access the next bit
}
return result; // Return the reversed 16-bit number
}
console.log(sixteen_bits_reverse(12345));
console.log(sixteen_bits_reverse(10));
console.log(sixteen_bits_reverse(5));
Output:
39948 20480 40960
Live Demo:
See the Pen javascript-basic-exercise-137 by w3resource (@w3resource) on CodePen.
Flowchart:
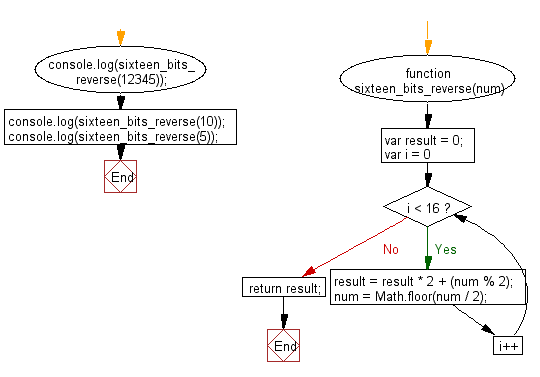
ES6 Version:
/**
* Function to reverse the bits of a number considering 16 bits
* @param {number} num - The input number
* @returns {number} - The result after reversing the bits (considering 16 bits)
*/
const sixteen_bits_reverse = (num) => {
let result = 0; // Variable to store the result after reversing bits, initialized to 0
for (let i = 0; i < 16; i++) {
result = result * 2 + (num % 2); // Multiplying the result by 2 and adding the last bit of 'num'
num = Math.floor(num / 2); // Dividing 'num' by 2 (integer division)
}
return result; // Returning the final result after reversing 16 bits
};
console.log(sixteen_bits_reverse(12345));
console.log(sixteen_bits_reverse(10));
console.log(sixteen_bits_reverse(5));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that reverses the bits of a 16-bit unsigned integer using bitwise manipulation techniques.
- Write a JavaScript function that converts a 16-bit number to binary, reverses its bit sequence, and converts it back to a decimal value.
- Write a JavaScript program that handles a 16-bit unsigned integer, reverses its bits, and returns the resulting integer with proper padding.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to test if a given integer is greater than 15 return the given number, otherwise return 15.
Next: JavaScript program to check whether it is possible to rearrange characters of a given string in such way that it will become equal to another given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.