JavaScript: Replace the first digit in a string (should contains at least digit) with $ character
JavaScript Basic: Exercise-136 with Solution
Replace First Digit in String with $
Write a JavaScript program to replace the first digit in a string (should have at least one digit) with the $ character.
Visual Presentation:
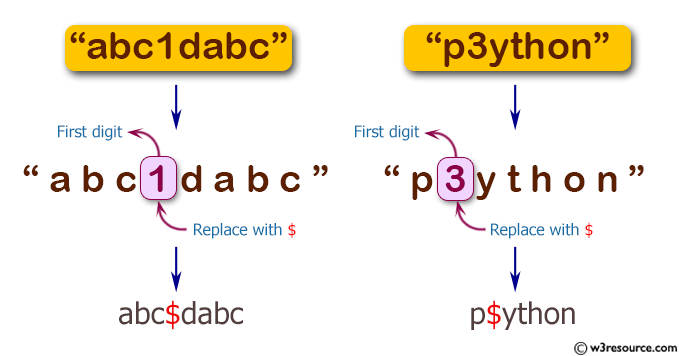
Sample Solution:
JavaScript Code:
Output:
abc$dabc p$ython ab$cabc
Live Demo:
Flowchart:
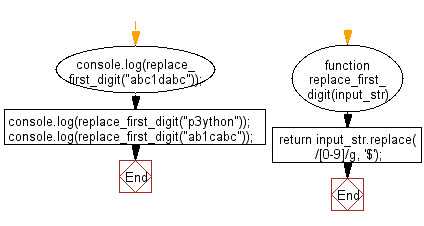
ES6 Version:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that locates the first digit in a string and replaces it with the '$' character, preserving the remaining characters.
- Write a JavaScript function that searches for the first occurrence of a numerical digit in a string and substitutes it with a dollar sign.
- Write a JavaScript program that scans a string for its first digit and replaces that digit with '$', ensuring the string contains at least one digit.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to remove all characters from a given string that appear more than once.
Next: JavaScript program to test if a given integer is greater than 15 return the given number, otherwise return 15.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.