JavaScript: Remove all characters from a given string that appear more than once
JavaScript Basic: Exercise-135 with Solution
Remove Characters Appearing More Than Once
Write a JavaScript program to remove all characters from a given string that appear more than once.
Visual Presentation:
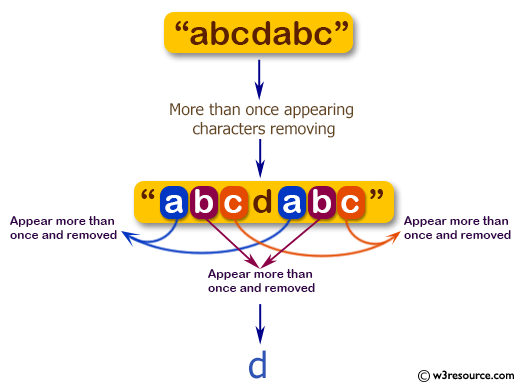
Sample Solution:
JavaScript Code:
/**
* Function to remove duplicate characters from a string
* @param {string} str - The input string
* @returns {string} - The string without duplicate characters
*/
function remove_duplicate_chars(str) {
// Split the string into an array of characters
var arr_char = str.split("");
var result_arr = [];
// Loop through each character of the array
for (var i = 0; i < arr_char.length; i++) {
// Check if the first and last occurrence of a character in the string are the same
if (str.indexOf(arr_char[i]) === str.lastIndexOf(arr_char[i]))
result_arr.push(arr_char[i]);
}
// Join the array of unique characters and return as a string
return result_arr.join("");
}
console.log(remove_duplicate_chars("abcdabc"));
console.log(remove_duplicate_chars("python"));
console.log(remove_duplicate_chars("abcabc"));
console.log(remove_duplicate_chars("1365451"));
Output:
d python 364
Live Demo:
See the Pen javascript-basic-exercise-135 by w3resource (@w3resource) on CodePen.
Flowchart:
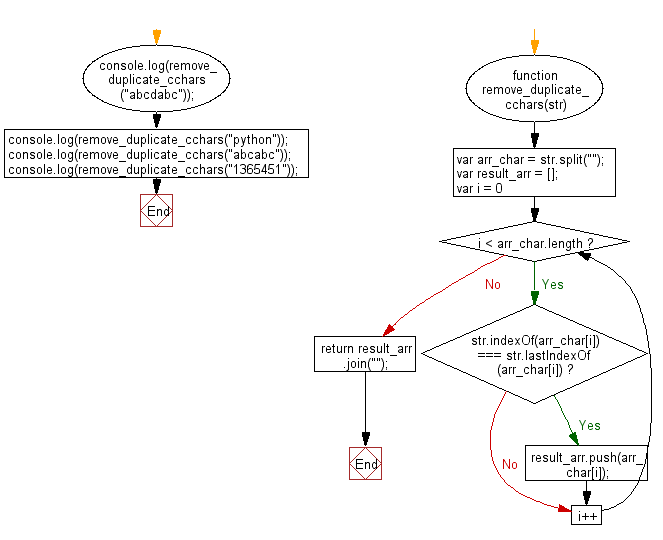
ES6 Version:
/**
* Function to remove duplicate characters from a string
* @param {string} str - The input string
* @returns {string} - The string without duplicate characters
*/
const remove_duplicate_chars = (str) => {
// Split the string into an array of characters
const arr_char = str.split("");
const result_arr = [];
// Loop through each character of the array
for (let i = 0; i < arr_char.length; i++) {
// Check if the first and last occurrence of a character in the string are the same
if (str.indexOf(arr_char[i]) === str.lastIndexOf(arr_char[i]))
result_arr.push(arr_char[i]);
}
// Join the array of unique characters and return as a string
return result_arr.join("");
}
console.log(remove_duplicate_chars("abcdabc"));
console.log(remove_duplicate_chars("python"));
console.log(remove_duplicate_chars("abcabc"));
console.log(remove_duplicate_chars("1365451"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes all characters from a string that occur more than once, leaving only unique characters.
- Write a JavaScript function that builds a new string by filtering out characters with multiple occurrences in the original string.
- Write a JavaScript program that analyzes the frequency of each character and constructs a new string containing only single-occurrence characters.
Go to:
PREV : Reverse Alphabetical Order of Lowercase Letters.
NEXT : Replace First Digit in String with $.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.