JavaScript: Change the characters (lower case) in a string where a turns into z, b turns into y, c turns into x, ..., n turns into m, m turns into n, ..., z turns into a
JavaScript Basic: Exercise-134 with Solution
Reverse Alphabetical Order of Lowercase Letters
Write a JavaScript program to change the characters (lower case) in a string where a turns into z, b turns into y, c turns into x, ..., n turns into m, m turns into n, ..., z turns into a.
Visual Presentation:
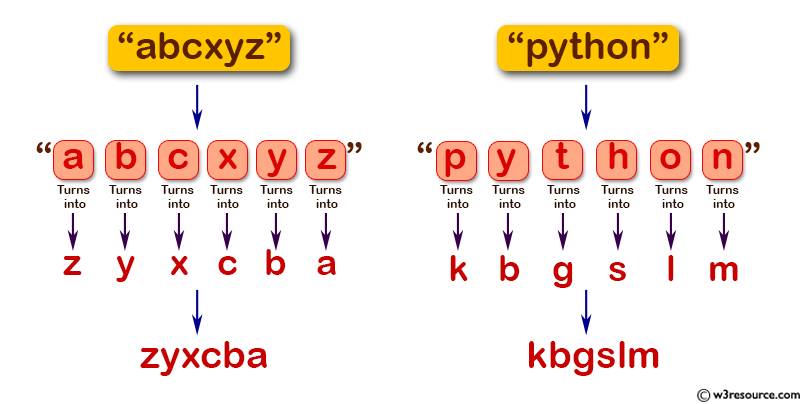
Sample Solution:
JavaScript Code:
// Function to change characters in a string based on specified logic
function change_char(str1) {
var result = []; // Initialize an empty array to store the resulting characters
for (var i = 0; i < str1.length; i++) {
var char_order = str1.charCodeAt(i) - 'a'.charCodeAt(0); // Get the character code and calculate its order relative to 'a'
var change_char = 25 - char_order + 'a'.charCodeAt(0); // Calculate the new character based on the order difference
result.push(String.fromCharCode(change_char)); // Convert the character code back to character and add it to the result array
}
return result.join(""); // Join the characters in the result array into a string and return
}
// Test cases
console.log(change_char("abcxyz")); // Output: "zyxcba"
console.log(change_char("python")); // Output: "kbgslm"
Output:
zyxcba kbgslm
Live Demo:
See the Pen javascript-basic-exercise-134 by w3resource (@w3resource) on CodePen.
Flowchart:
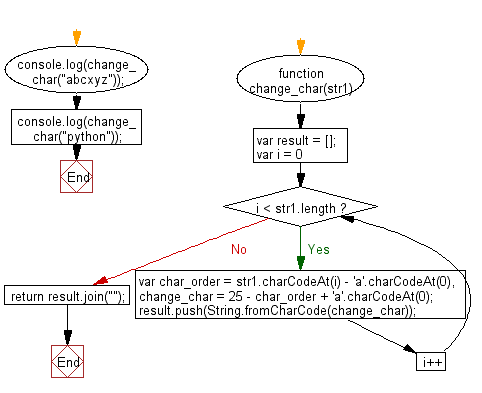
ES6 Version:
/**
* Function to change characters in a string based on specified logic.
* @param {string} str1 - The input string
* @returns {string} - The modified string
*/
const change_char = (str1) => {
const result = [];
for (let i = 0; i < str1.length; i++) {
const char_order = str1.charCodeAt(i) - 'a'.charCodeAt(0);
const change_char = 25 - char_order + 'a'.charCodeAt(0);
result.push(String.fromCharCode(change_char));
}
return result.join("");
};
console.log(change_char("abcxyz"));
console.log(change_char("python"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given fraction is proper or not.
Next: JavaScript program to remove all characters from a given string that appear more than once.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics