JavaScript: Check whether a given fraction is proper or not
JavaScript Basic: Exercise-133 with Solution
Check if Fraction is Proper
Write a JavaScript program to check whether a given fraction is proper or not.
Note: There are two types of common fractions, proper or improper. When the numerator and the denominator are both positive, the fraction is called proper if the numerator is less than the denominator, and improper otherwise.
Sample Solution:
JavaScript Code:
// Function to determine if the fraction is proper or improper
function proper_improper_test(num) {
// Check if the absolute value of the division is less than 1
return Math.abs(num[0] / num[1]) < 1
? "Proper fraction." // Return 'Proper fraction.' if the condition is true
: "Improper fraction."; // Otherwise, return 'Improper fraction.'
}
// Test cases
console.log(proper_improper_test([12, 300])); // Output: Proper fraction.
console.log(proper_improper_test([2, 4])); // Output: Proper fraction.
console.log(proper_improper_test([103, 3])); // Output: Improper fraction.
console.log(proper_improper_test([104, 2])); // Output: Improper fraction.
console.log(proper_improper_test([5, 40])); // Output: Proper fraction.
Output:
Proper fraction. Proper fraction. Improper fraction. Improper fraction. Proper fraction.
Live Demo:
See the Pen javascript-basic-exercise-133 by w3resource (@w3resource) on CodePen.
Flowchart:
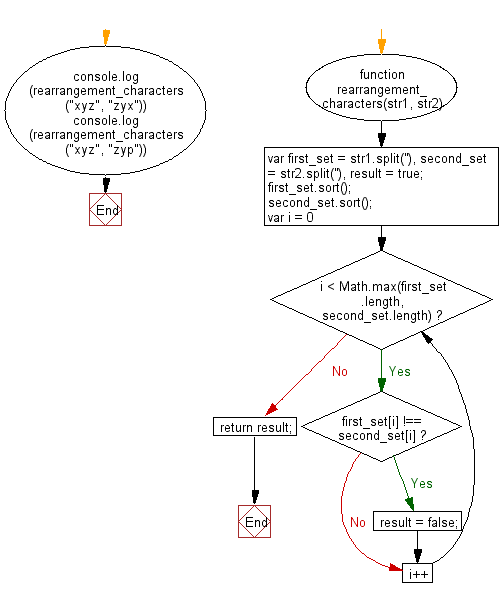
ES6 Version:
// Function to determine if the fraction is proper or improper
const proper_improper_test = (num) => {
// Check if the absolute value of the division is less than 1
return Math.abs(num[0] / num[1]) < 1
? "Proper fraction." // Return 'Proper fraction.' if the condition is true
: "Improper fraction."; // Otherwise, return 'Improper fraction.'
};
// Test cases
console.log(proper_improper_test([12, 300])); // Output: Proper fraction.
console.log(proper_improper_test([2, 4])); // Output: Proper fraction.
console.log(proper_improper_test([103, 3])); // Output: Improper fraction.
console.log(proper_improper_test([104, 2])); // Output: Improper fraction.
console.log(proper_improper_test([5, 40])); // Output: Proper fraction.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks whether a fraction (two positive integers) is proper by comparing the numerator and denominator.
- Write a JavaScript function that returns true if the numerator is less than the denominator, and false otherwise.
- Write a JavaScript program that validates a fraction’s properness and handles edge cases such as zero denominators.
Go to:
PREV : Find Distinct Prime Factors of Integer.
NEXT : Reverse Alphabetical Order of Lowercase Letters.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.