JavaScript: Find all distinct prime factors of a given integer
JavaScript Basic: Exercise-132 with Solution
Find Distinct Prime Factors of Integer
Write a JavaScript program to find all distinct prime factors of a given integer
Visual Presentation:
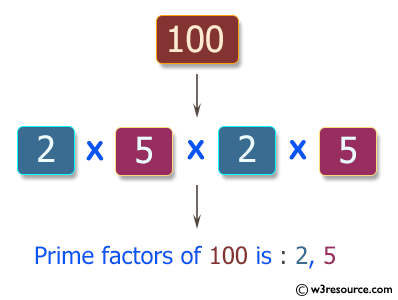
Sample Solution:
JavaScript Code:
// Function to find prime factors of a number
function prime_factors(num) {
// Function to check if a number is prime
function is_prime(num) {
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false; // If the number is divisible by any number other than 1 and itself, it's not a prime
}
return true; // Return true if the number is prime
}
const result = []; // Initialize an empty array to store prime factors
// Loop through numbers from 2 to 'num'
for (let i = 2; i <= num; i++) {
// While 'i' is a prime factor and divides 'num' evenly
while (is_prime(i) && num % i === 0) {
if (!result.includes(i)) result.push(i); // Add 'i' to the result array if it's not already present
num /= i; // Divide 'num' by 'i'
}
}
return result; // Return the array containing prime factors
}
// Test cases
console.log(prime_factors(100)); // Output: [2, 5]
console.log(prime_factors(101)); // Output: [101]
console.log(prime_factors(103)); // Output: [103]
console.log(prime_factors(104)); // Output: [2, 13]
console.log(prime_factors(105)); // Output: [3, 5, 7]
Output:
[2,5] [101] [103] [2,13] [3,5,7]
Live Demo:
See the Pen javascript-basic-exercise-132 by w3resource (@w3resource) on CodePen.
Flowchart:
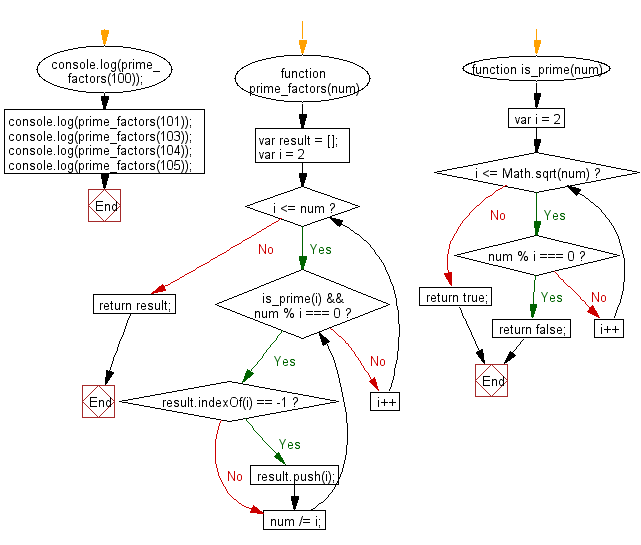
ES6 Version:
function prime_factors(num) {
function is_prime(num) {
for (let i = 2; i <= Math.sqrt(num); i++)
{
if (num % i === 0) return false;
}
return true;
}
const result = [];
for (let i = 2; i <= num; i++)
{
while (is_prime(i) && num % i === 0)
{
if (!result.includes(i)) result.push(i);
num /= i;
}
}
return result;
}
console.log(prime_factors(100));
console.log(prime_factors(101));
console.log(prime_factors(103));
console.log(prime_factors(104));
console.log(prime_factors(105));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes all prime factors of a given integer and returns only the distinct ones.
- Write a JavaScript function that factors an integer into its prime components and eliminates duplicate factors in the result.
- Write a JavaScript program that iterates through potential divisors to determine the unique prime factors of an input number.
Go to:
PREV : Create Prefix Sum Array.
NEXT : Check if Fraction is Proper.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.