JavaScript: Find the number of even digits in a given integer
JavaScript Basic: Exercise-130 with Solution
Count Even Digits in Integer
Write a JavaScript program to find the number of even digits in a given integer.
Visual Presentation:
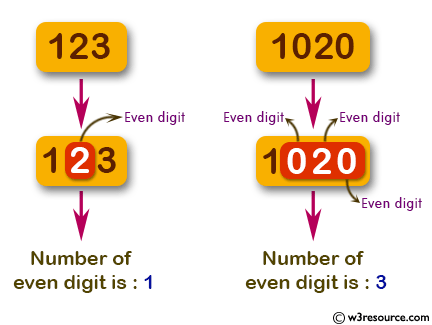
Sample Solution:
JavaScript Code:
// Function to count the number of even digits in a given number
function even_digits(num) {
var ctr = 0;
// Loop until 'num' becomes 0
while (num) {
// Increment 'ctr' if the last digit of 'num' is even
ctr += num % 2 === 0;
// Remove the last digit by integer division
num = Math.floor(num / 10);
}
return ctr; // Return the count of even digits
}
// Test cases
console.log(even_digits(123)); // Output: 1
console.log(even_digits(1020)); // Output: 3
console.log(even_digits(102)); // Output: 2
Output:
1 3 2
Live Demo:
See the Pen javascript-basic-exercise-130 by w3resource (@w3resource) on CodePen.
Flowchart:
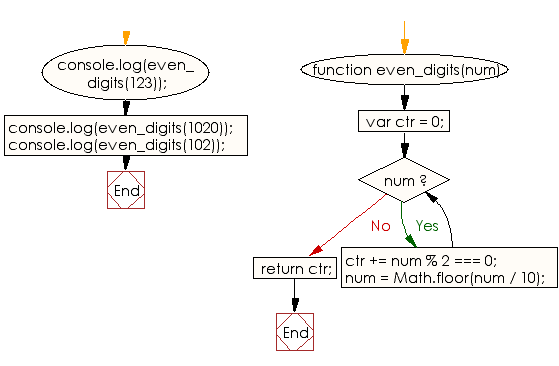
ES6 Version:
// Function to count the number of even digits in a given number
const even_digits = (num) => {
let ctr = 0;
// Loop until 'num' becomes 0
while (num) {
// Increment 'ctr' if the last digit of 'num' is even
ctr += num % 2 === 0;
// Remove the last digit by integer division
num = Math.floor(num / 10);
}
return ctr; // Return the count of even digits
};
// Test cases
console.log(even_digits(123)); // Output: 1
console.log(even_digits(1020)); // Output: 3
console.log(even_digits(102)); // Output: 2
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts an integer to a string and counts the number of even digits within it.
- Write a JavaScript function that iterates over each digit of a number and returns the count of digits that are even using modulus operations.
- Write a JavaScript program that processes a given integer and outputs the total number of even digits found in it.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the smallest prime number strictly greater than a given number.
Next: JavaScript program to create an array of prefix sums of the given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.