JavaScript: Find the smallest prime number strictly greater than a given number
JavaScript Basic: Exercise-129 with Solution
Find Smallest Prime > Value
Write a JavaScript program to find the smallest prime number strictly greater than a given number.
Visual Presentation:
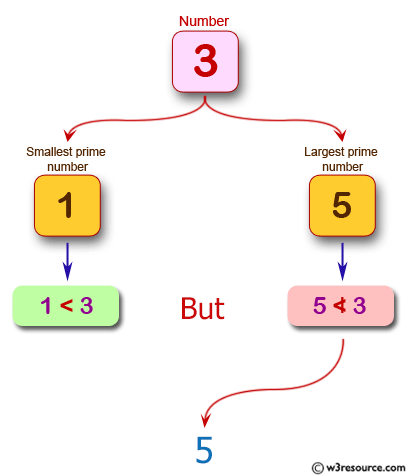
Sample Solution:
JavaScript Code:
// Function to find the next prime number after a given number
function next_Prime_num(num) {
// Start checking from the next number after 'num'
for (var i = num + 1;; i++) {
var isPrime = true;
// Check divisibility from 2 up to the square root of the number
for (var d = 2; d * d <= i; d++) {
// If 'i' is divisible by 'd', it's not a prime number
if (i % d === 0) {
isPrime = false;
break;
}
}
// If 'isPrime' is still true, return 'i' (it's a prime number)
if (isPrime) {
return i;
}
}
}
// Test cases
console.log(next_Prime_num(3)); // Output: 5
console.log(next_Prime_num(17)); // Output: 19
Output:
5 19
Live Demo:
See the Pen javascript-basic-exercise-129 by w3resource (@w3resource) on CodePen.
Flowchart:
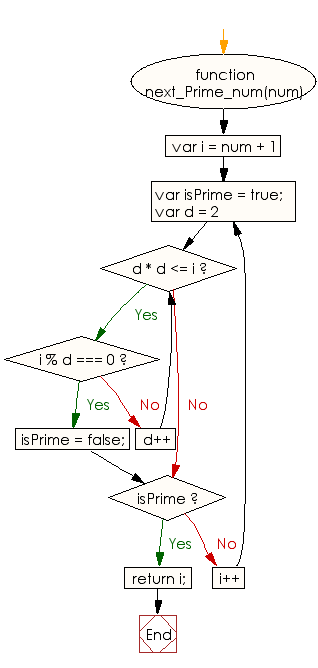
ES6 Version:
// Function to find the next prime number after a given number
const next_Prime_num = (num) => {
// Start checking from the next number after 'num'
for (let i = num + 1;; i++) {
let isPrime = true;
// Check divisibility from 2 up to the square root of the number
for (let d = 2; d * d <= i; d++) {
// If 'i' is divisible by 'd', it's not a prime number
if (i % d === 0) {
isPrime = false;
break;
}
}
// If 'isPrime' is still true, return 'i' (it's a prime number)
if (isPrime) {
return i;
}
}
}
// Test cases
console.log(next_Prime_num(3)); // Output: 5
console.log(next_Prime_num(17)); // Output: 19
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the smallest prime number strictly greater than a given number by iterating and testing for primality.
- Write a JavaScript function that checks each subsequent number for prime status and returns the first prime found.
- Write a JavaScript program that efficiently finds the next prime number after a given integer, including optimizations for large inputs.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the smallest round number that is not less than a given value.
Next: JavaScript program to find the number of even digits in a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.