JavaScript: Get the largest even number from an array of integers
JavaScript Basic: Exercise-126 with Solution
Find Largest Even Number in Array
Write a JavaScript program to get the largest even number from an array of integers.
Visual Presentation:
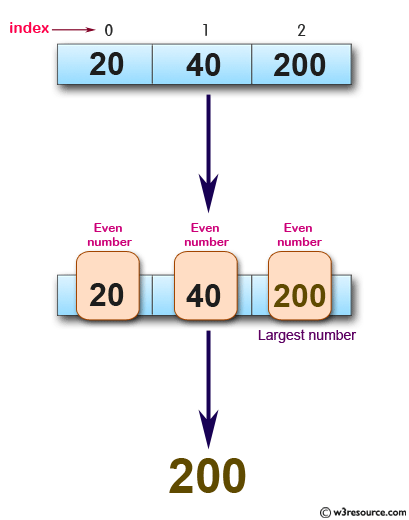
Sample Solution:
JavaScript Code:
// Function to find the maximum even number in an array
function max_even(arra) {
// Sort the array in descending order
arra.sort((x, y) => y - x);
// Loop through the array
for (var i = 0; i < arra.length; i++) {
// Check if the current number is even
if (arra[i] % 2 == 0)
return arra[i]; // If even, return the number
}
}
// Testing the function with different arrays
console.log(max_even([20, 40, 200])); // Output: 200 (The maximum even number in the array)
console.log(max_even([20, 40, 200, 301])); // Output: 200 (The maximum even number in the array)
Output:
200 200
Live Demo:
See the Pen javascript-basic-exercise-126 by w3resource (@w3resource) on CodePen.
Flowchart:
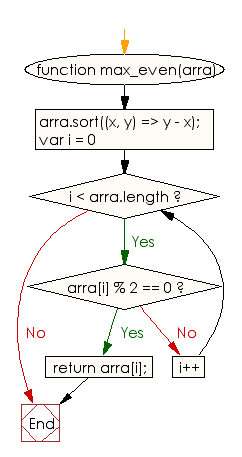
ES6 Version:
// Function to find the maximum even number in an array
const max_even = (arra) => {
// Sort the array in descending order
arra.sort((x, y) => y - x);
// Loop through the array
for (let i = 0; i < arra.length; i++) {
// Check if the current number is even
if (arra[i] % 2 == 0)
return arra[i]; // If even, return the number
}
}
// Testing the function with different arrays
console.log(max_even([20, 40, 200])); // Output: 200 (The maximum even number in the array)
console.log(max_even([20, 40, 200, 301])); // Output: 200 (The maximum even number in the array)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that filters out odd numbers from an array and returns the largest even number.
- Write a JavaScript function that iterates through an array to identify and return the maximum even integer, handling cases with no even numbers.
- Write a JavaScript program that uses array methods to extract even numbers and then determine the largest among them.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the longest string from a given array.
Next: JavaScript program to reverse the order of the bits in a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.