JavaScript: Find the longest string from a given array
JavaScript Basic: Exercise-125 with Solution
Find Longest String in Array
Write a JavaScript program to find the longest string in a given array.
Visual Presentation:
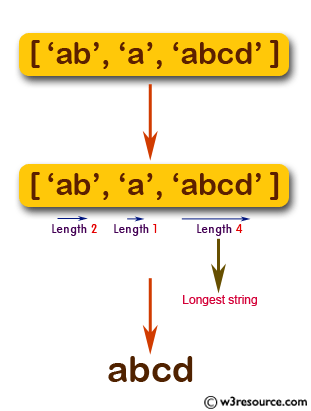
Sample Solution:
JavaScript Code:
// Function to find the longest string in an array
function longest_str_in_array(arra) {
var max_str = arra[0].length; // Initialize max_str with the length of the first string
var ans = arra[0]; // Initialize ans with the first string
// Loop through the array starting from the second element
for (var i = 1; i < arra.length; i++) {
var maxi = arra[i].length; // Get the length of the current string
// Compare the length of the current string with max_str
if (maxi > max_str) {
ans = arra[i]; // If current string is longer, update ans
max_str = maxi; // Update max_str with the new maximum length
}
}
return ans; // Return the longest string found
}
// Testing the function with different arrays
console.log(longest_str_in_array(["ab", "a", "abcd"])); // Output: "abcd" (Longest string in the array)
console.log(longest_str_in_array(["ab", "ab", "ab"])); // Output: "ab" (All strings are of equal length)
Output:
abcd ab
Live Demo:
See the Pen javascript-basic-exercise-125 by w3resource (@w3resource) on CodePen.
Flowchart:
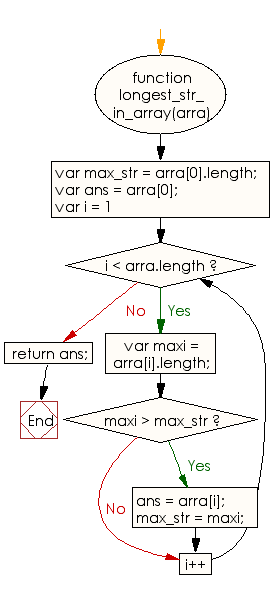
ES6 Version:
// Function to find the longest string in an array
const longest_str_in_array = (arra) => {
let max_str = arra[0].length; // Initialize max_str with the length of the first string
let ans = arra[0]; // Initialize ans with the first string
// Loop through the array starting from the second element
for (let i = 1; i < arra.length; i++) {
let maxi = arra[i].length; // Get the length of the current string
// Compare the length of the current string with max_str
if (maxi > max_str) {
ans = arra[i]; // If current string is longer, update ans
max_str = maxi; // Update max_str with the new maximum length
}
}
return ans; // Return the longest string found
}
// Testing the function with different arrays
console.log(longest_str_in_array(["ab", "a", "abcd"])); // Output: "abcd" (Longest string in the array)
console.log(longest_str_in_array(["ab", "ab", "ab"])); // Output: "ab" (All strings are of equal length)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create the value of NOR of two given booleans.
Next: JavaScript program to get the largest even number from an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics