JavaScript: Find whether the members of a given array of integers is a permutation of numbers from 1 to a given integer
JavaScript Basic: Exercise-123 with Solution
Check if Array is Permutation of Numbers 1 to n
Write a JavaScript program to find out if the members of a given array of integers are a permutation of numbers from 1 to a given integer.
Visual Presentation:
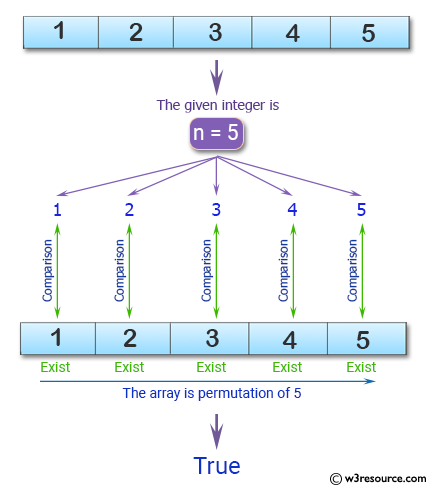
Sample Solution:
JavaScript Code:
// Function to check if input array is a permutation of [1, 2, 3, ..., n]
function is_permutation(input_arr, n) {
// Loop through each element from 1 to n
for (var i = 0; i < n; i++) {
// Check if the current element is not found in the input array
if (input_arr.indexOf(i + 1) < 0) {
return false; // If not found, it's not a permutation
}
}
return true; // If all elements are found, it's a permutation
}
// Test cases
console.log(is_permutation([1, 2, 3, 4, 5], 5)); // Output: true (permutation of [1, 2, 3, 4, 5])
console.log(is_permutation([1, 2, 3, 5], 5)); // Output: false (not a permutation of [1, 2, 3, 4, 5])
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-123 by w3resource (@w3resource) on CodePen.
Flowchart:
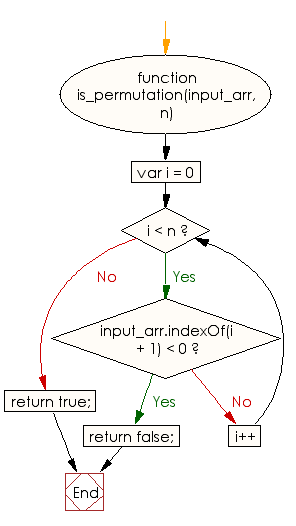
ES6 Version:
// Function to check if input array is a permutation of [1, 2, 3, ..., n]
const is_permutation = (input_arr, n) => {
// Loop through each element from 1 to n
for (let i = 0; i < n; i++) {
// Check if the current element is not found in the input array
if (input_arr.indexOf(i + 1) < 0) {
return false; // If not found, it's not a permutation
}
}
return true; // If all elements are found, it's a permutation
}
// Test cases
console.log(is_permutation([1, 2, 3, 4, 5], 5)); // Output: true (permutation of [1, 2, 3, 4, 5])
console.log(is_permutation([1, 2, 3, 5], 5)); // Output: false (not a permutation of [1, 2, 3, 4, 5])
For more Practice: Solve these Related Problems:
- Write a JavaScript program that verifies if an array is a permutation of numbers from 1 to n by checking for duplicates and missing elements.
- Write a JavaScript function that sorts an array and compares it with an ideal sequence from 1 to n to confirm it is a valid permutation.
- Write a JavaScript program that uses a frequency counter to determine if the elements of an array form a complete permutation of numbers 1 to n.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given array of integers represents either a strictly increasing or a strictly decreasing sequence.
Next: JavaScript program to create the value of NOR of two given booleans.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.