JavaScript: Check whether a given array of integers represents either a strictly increasing or a strictly decreasing sequence
JavaScript Basic: Exercise-122 with Solution
Check if Array is Strictly Increasing/Decreasing
Write a JavaScript program to check whether a given array of integers represents a strictly increasing or decreasing sequence.
Visual Presentation:
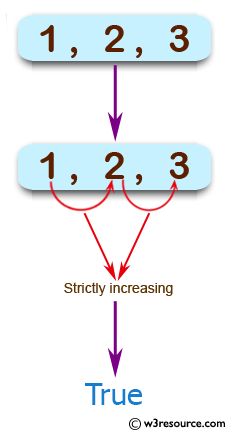
Sample Solution:
JavaScript Code:
// Function to check if a sequence of numbers is monotonous
function is_monotonous(num) {
// If the sequence has only one element, it's considered monotonous
if (num.length === 1) {
return true;
}
// Calculate the direction of the sequence
var num_direction = num[1] - num[0];
// Check for non-monotonic behavior
for (var i = 0; i < num.length - 1; i++) {
// If the product of direction and the difference between elements is not positive, it's non-monotonic
if (num_direction * (num[i + 1] - num[i]) <= 0) {
return false;
}
}
// If the loop completes, the sequence is monotonic
return true;
}
// Test cases
console.log(is_monotonous([1, 2, 3])); // Output: true (Monotonously increasing)
console.log(is_monotonous([1, 2, 2])); // Output: false (Not strictly increasing)
console.log(is_monotonous([-3, -2, -1])); // Output: true (Monotonously increasing)
Output:
true false true
Live Demo:
See the Pen javascript-basic-exercise-122 by w3resource (@w3resource) on CodePen.
Flowchart:
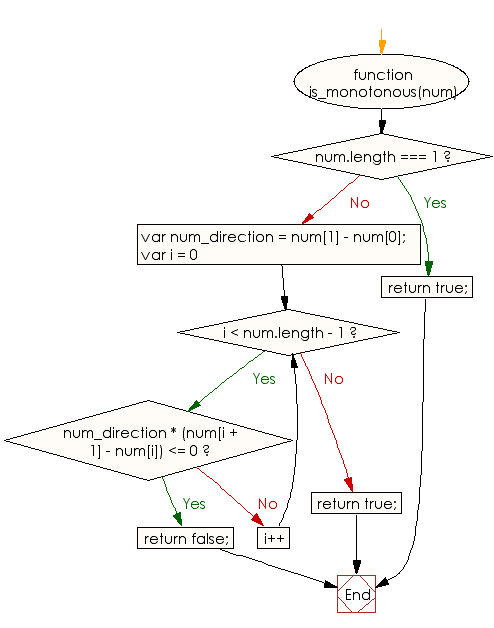
ES6 Version:
// Function to check if a sequence of numbers is monotonous
const is_monotonous = (num) => {
// If the sequence has only one element, it's considered monotonous
if (num.length === 1) {
return true;
}
// Calculate the direction of the sequence
const num_direction = num[1] - num[0];
// Check for non-monotonic behavior
for (let i = 0; i < num.length - 1; i++) {
// If the product of direction and the difference between elements is not positive, it's non-monotonic
if (num_direction * (num[i + 1] - num[i]) <= 0) {
return false;
}
}
// If the loop completes, the sequence is monotonic
return true;
};
// Test cases
console.log(is_monotonous([1, 2, 3])); // Output: true (Monotonously increasing)
console.log(is_monotonous([1, 2, 2])); // Output: false (Not strictly increasing)
console.log(is_monotonous([-3, -2, -1])); // Output: true (Monotonously increasing)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if a given matrix is lower triangular or not.
Next: JavaScript program to find if the members of an given array of integers is a permutation of numbers from 1 to a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics