JavaScript: Check whether a given matrix is lower triangular or not
JavaScript Basic: Exercise-121 with Solution
Check if Matrix is Lower Triangular
Write a JavaScript program to check whether a given matrix is lower triangular or not.
Note: A square matrix is called lower triangular if all the entries above the main diagonal are zero.
Sample Solution:
JavaScript Code:
// Function to check if a matrix is a lower triangular matrix
function lower_triangular_matrix(user_matrix) {
for (var i = 0; i < user_matrix.length; i++) {
for (var j = 0; j < user_matrix[0].length; j++) {
// If element is above the main diagonal and not equal to zero, return false
if (j > i && user_matrix[i][j] !== 0) {
return false;
}
}
}
return true; // Matrix is lower triangular
}
// Test cases
console.log(lower_triangular_matrix([[1, 0, 0],[2, 0, 0], [0, 3, 3]])); // Output: true (Lower triangular matrix)
console.log(lower_triangular_matrix([[1, 0, 1],[2, 0, 0], [0, 3, 3]])); // Output: false (Not a lower triangular matrix)
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-121 by w3resource (@w3resource) on CodePen.
Flowchart:
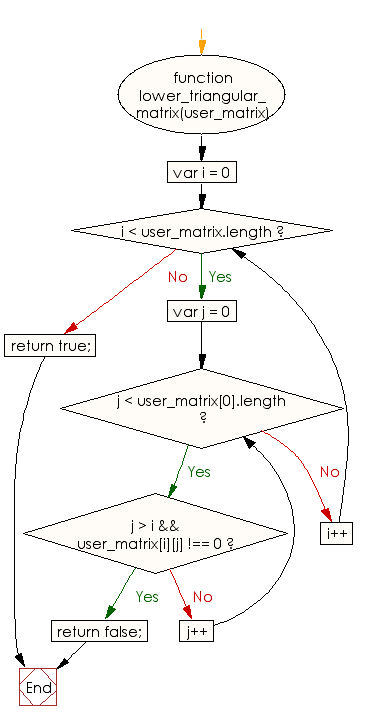
ES6 Version:
// Function to check if a matrix is a lower triangular matrix
const lower_triangular_matrix = (user_matrix) => {
for (let i = 0; i < user_matrix.length; i++) {
for (let j = 0; j < user_matrix[0].length; j++) {
// If element is above the main diagonal and not equal to zero, return false
if (j > i && user_matrix[i][j] !== 0) {
return false;
}
}
}
return true; // Matrix is lower triangular
};
// Test cases
console.log(lower_triangular_matrix([[1, 0, 0],[2, 0, 0], [0, 3, 3]])); // Output: true (Lower triangular matrix)
console.log(lower_triangular_matrix([[1, 0, 1],[2, 0, 0], [0, 3, 3]])); // Output: false (Not a lower triangular matrix)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a square matrix is lower triangular by confirming all elements above the main diagonal are zero.
- Write a JavaScript function that iterates over a matrix and returns true if every element with a column index greater than its row index is zero.
- Write a JavaScript program that validates whether a matrix is lower triangular by comparing each element's row and column indices.
Go to:
PREV : Check if Point is Inside Circle.
NEXT : Check if Array is Strictly Increasing/Decreasing.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.