JavaScript: Check whether a given integer has an increasing digits sequence
JavaScript Basic: Exercise-119 with Solution
Check if Digits in Integer Are Increasing
Write a JavaScript program to check if a given integer has an increasing digit sequence.
Visual Presentation:
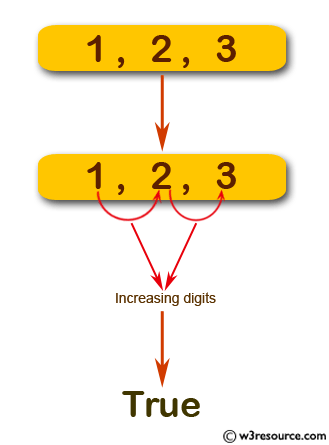
Sample Solution:
JavaScript Code:
// Function to check if the digits of a number form an increasing sequence
function is_increasing_digits_Sequence(num) {
var arr_num = ('' + num).split(''); // Convert the number to a string and split it into an array of digits
// Loop through the array of digits
for (var i = 0; i < arr_num.length - 1; i++) {
// Check if the current digit is greater than or equal to the next digit
if (parseInt(arr_num[i]) >= parseInt(arr_num[i + 1])) {
return false; // If the sequence is not increasing, return false
}
}
return true; // If the sequence is increasing, return true
}
// Test cases
console.log(is_increasing_digits_Sequence(123)); // Output: true (Each digit forms an increasing sequence)
console.log(is_increasing_digits_Sequence(1223)); // Output: false (Digits are not in strictly increasing order)
console.log(is_increasing_digits_Sequence(45677)); // Output: false (Digits are not in strictly increasing order)
Output:
true false false
Live Demo:
See the Pen javascript-basic-exercise-119 by w3resource (@w3resource) on CodePen.
Flowchart:
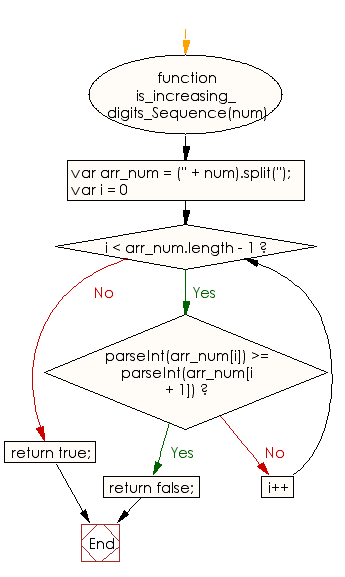
ES6 Version:
// Function to check if the digits of a number form an increasing sequence
const is_increasing_digits_Sequence = (num) => {
const arr_num = `${num}`.split(''); // Convert the number to a string and split it into an array of digits
// Loop through the array of digits
for (let i = 0; i < arr_num.length - 1; i++) {
// Check if the current digit is greater than or equal to the next digit
if (parseInt(arr_num[i]) >= parseInt(arr_num[i + 1])) {
return false; // If the sequence is not increasing, return false
}
}
return true; // If the sequence is increasing, return true
};
// Test cases
console.log(is_increasing_digits_Sequence(123)); // Output: true (Each digit forms an increasing sequence)
console.log(is_increasing_digits_Sequence(1223)); // Output: false (Digits are not in strictly increasing order)
console.log(is_increasing_digits_Sequence(45677)); // Output: false (Digits are not in strictly increasing order)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given number is in a given range.
Next: JavaScript program to check whether a point lies strictly inside a given circle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics