JavaScript: Check whether a given number is in a given range
JavaScript Basic: Exercise-118 with Solution
Check if Number is in Range
Write a JavaScript program to check whether a given number is in a given range.
Visual Presentation:
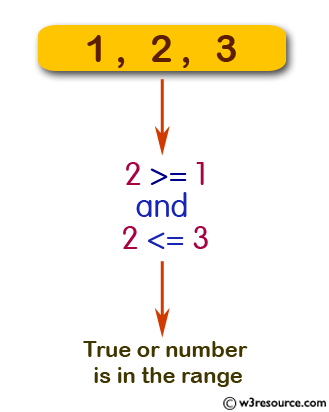
Sample Solution:
JavaScript Code:
// Function to check if 'y' lies within the range of 'x' and 'z'
function is_inrange(x, y, z)
{
return y >= x && y <= z; // Returns true if 'y' is between 'x' and 'z' (inclusive)
}
console.log(is_inrange(1,2,3)); // Output: true (2 is in the range between 1 and 3)
console.log(is_inrange(1,2,-3)); // Output: false (2 is not in the range between 1 and -3)
console.log(is_inrange(1.1,1.2,1.3)); // Output: true (1.2 is in the range between 1.1 and 1.3)
Output:
true false true
Live Demo:
See the Pen javascript-basic-exercise-118 by w3resource (@w3resource) on CodePen.
Flowchart:
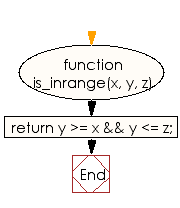
ES6 Version:
// Function to check if 'y' lies within the range of 'x' and 'z'
const is_inrange = (x, y, z) => {
return y >= x && y <= z; // Returns true if 'y' is between 'x' and 'z' (inclusive)
};
console.log(is_inrange(1,2,3)); // Output: true (2 is in the range between 1 and 3)
console.log(is_inrange(1,2,-3)); // Output: false (2 is not in the range between 1 and -3)
console.log(is_inrange(1.1,1.2,1.3)); // Output: true (1.2 is in the range between 1.1 and 1.3)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines if a given number falls within a specified inclusive range using logical operators.
- Write a JavaScript function that accepts a number and range boundaries and returns a boolean indicating whether the number is within range.
- Write a JavaScript program that validates an input number against a dynamic range provided by the user and outputs an appropriate message.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given matrix is an identity matrix.
Next: JavaScript program to check whether a given integer has an increasing digits sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.