JavaScript: Find all the possible options to replace the hash in a string
JavaScript Basic: Exercise-116 with Solution
Replace Hash in String to Make Divisible by 3
Write a JavaScript program to find all the possible options to replace the hash in a string (Consists of digits and one hash (#)) with a digit to produce an integer divisible by 3.
For a string "2*0", the output should be : ["210", "240", "270"]
Sample Solution:
JavaScript Code:
// Function to generate strings by replacing '#' in the input with digits making the resulting number divisible by 3
function is_divisible_by3(mask_str) {
var digitSum = 0, // Variable to hold the sum of digits in the mask string
left = '0'.charCodeAt(), // ASCII code of '0'
right = '9'.charCodeAt(), // ASCII code of '9'
result = [], // Array to store the resulting strings
mask_data = mask_str.split(''), // Split the input mask string into an array
hash_pos = -1; // Position of the '#' character
// Loop through the characters in the mask string array
for (var i = 0; i < mask_data.length; i++) {
if (left <= mask_data[i].charCodeAt() && mask_data[i].charCodeAt() <= right) {
digitSum += mask_data[i].charCodeAt() - left; // Calculate digit sum if character is a digit
} else {
hash_pos = i; // Store the position of '#' character
}
}
// Loop through numbers 0 to 9 to replace '#' with digits making the number divisible by 3
for (var i = 0; i < 10; i++) {
if ((digitSum + i) % 3 === 0) { // Check if the sum of digits with the new digit is divisible by 3
mask_data[hash_pos] = String.fromCharCode(left + i); // Replace '#' with the new digit
result.push(mask_data.join('')); // Push the modified mask string to the result array
}
}
return result; // Return the array containing strings with the '#' replaced by digits
}
// Testing the function with sample inputs
console.log(is_divisible_by3("2#0")); // Output: [ '210', '240', '270' ]
console.log(is_divisible_by3("4#2")); // Output: [ '402', '432', '462', '492' ]
Output:
["210","240","270"] ["402","432","462","492"]
Live Demo:
See the Pen javascript-basic-exercise-116 by w3resource (@w3resource) on CodePen.
Flowchart:
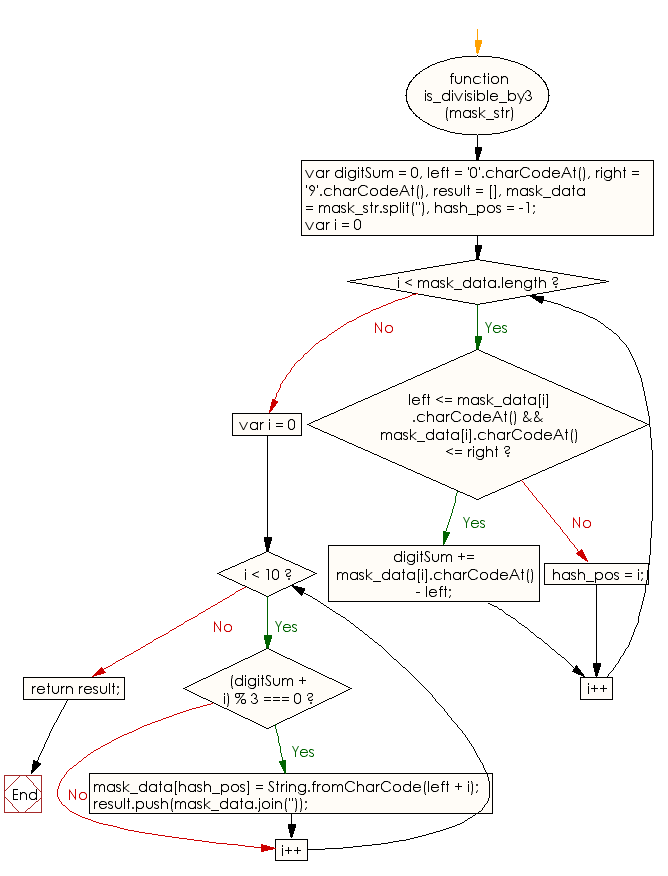
ES6 Version:
// Function to generate strings by replacing '#' in the input with digits making the resulting number divisible by 3
const is_divisible_by3 = (mask_str) => {
let digitSum = 0; // Variable to hold the sum of digits in the mask string
const left = '0'.charCodeAt(); // ASCII code of '0'
const right = '9'.charCodeAt(); // ASCII code of '9'
const result = []; // Array to store the resulting strings
const mask_data = [...mask_str]; // Convert the input mask string to an array
let hash_pos = -1; // Position of the '#' character
// Loop through the characters in the mask string array
for (let i = 0; i < mask_data.length; i++) {
if (left <= mask_data[i].charCodeAt() && mask_data[i].charCodeAt() <= right) {
digitSum += mask_data[i].charCodeAt() - left; // Calculate digit sum if character is a digit
} else {
hash_pos = i; // Store the position of '#' character
}
}
// Loop through numbers 0 to 9 to replace '#' with digits making the number divisible by 3
for (let i = 0; i < 10; i++) {
if ((digitSum + i) % 3 === 0) { // Check if the sum of digits with the new digit is divisible by 3
mask_data[hash_pos] = String.fromCharCode(left + i); // Replace '#' with the new digit
result.push(mask_data.join('')); // Push the modified mask string to the result array
}
}
return result; // Return the array containing strings with the '#' replaced by digits
};
// Testing the function with sample inputs
console.log(is_divisible_by3("2#0")); // Output: [ '210', '240', '270' ]
console.log(is_divisible_by3("4#2")); // Output: [ '402', '432', '462', '492' ]
For more Practice: Solve these Related Problems:
- Write a JavaScript program that replaces a hash in a numeric string with digits 0–9 to yield a number divisible by 3.
- Write a JavaScript function that iterates through possible digit replacements for a hash placeholder and returns valid combinations divisible by 3.
- Write a JavaScript program that uses modular arithmetic to test each replacement for a hash in a string and outputs all options yielding divisibility by 3.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a matrix is a diagonal matrix or not.
Next: JavaScript program to check if a given matrix is an identity matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.