JavaScript: Calculate the sum of n + n/2 + n/4 + n/8 + ....
JavaScript Basic: Exercise-113 with Solution
Sum n + n/2 + n/4 + ...
Write a JavaScript program to calculate the sum of n + n/2 + n/4 + n/8 + .... where n is a positive integer and all divisions are integers.
Visual Presentation:
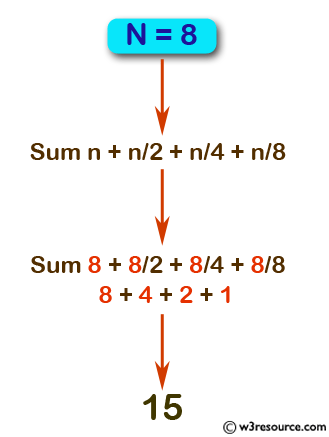
Sample Solution:
JavaScript Code:
// Function to calculate the sum of integers from 1 to num using bitwise operation
function int_sum(num) {
var s_sum = 0; // Initialize the sum variable
while (num > 0) { // Loop until num is greater than 0
s_sum += num; // Add num to the sum
num = Math.floor(num / 2); // Divide num by 2 using floor division
}
return s_sum; // Return the sum of integers from 1 to num
}
// Examples to calculate the sum of integers from 1 to the given number
console.log(int_sum(8));
console.log(int_sum(9));
console.log(int_sum(26));
Output:
15 16 49
Live Demo:
See the Pen javascript-basic-exercise-113 by w3resource (@w3resource) on CodePen.
Flowchart:
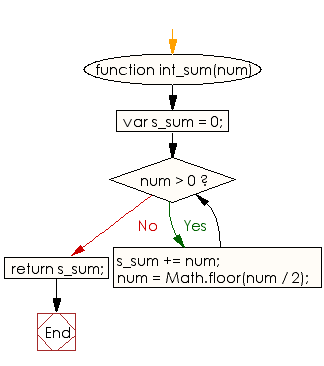
ES6 Version:
// Function to calculate the sum of integers from 1 to num using bitwise operation
const int_sum = (num) => {
let s_sum = 0; // Initialize the sum variable
while (num > 0) { // Loop until num is greater than 0
s_sum += num; // Add num to the sum
num = Math.floor(num / 2); // Divide num by 2 using floor division
}
return s_sum; // Return the sum of integers from 1 to num
}
// Examples to calculate the sum of integers from 1 to the given number
console.log(int_sum(8));
console.log(int_sum(9));
console.log(int_sum(26));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the number of trailing zeros in the decimal representation of the factorial of a given number.
Next: JavaScript program to check whether a given string represents a correct sentence or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics