JavaScript: Find the number of trailing zeros in the decimal representation of the factorial of a given number
JavaScript Basic: Exercise-112 with Solution
Count Trailing Zeros in Factorial
Write a JavaScript program to find the number of trailing zeros in the decimal representation of the factorial of a given number.
Visual Presentation:
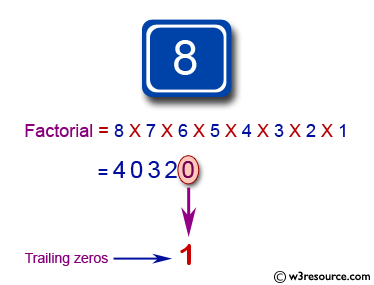
Sample Solution:
JavaScript Code:
// Function to count trailing zeros in the factorial of a number
function trailing_zeros_factorial(n) {
var result = 0; // Initialize the count of trailing zeros to zero
for (var i = 5; i <= n; i += 5) { // Loop to calculate the factorial
var num = i; // Store the current number
while (num % 5 === 0) { // Check if the number is divisible by 5
num /= 5; // Divide the number by 5
result++; // Increment the count of trailing zeros
}
}
return result; // Return the total count of trailing zeros in the factorial of n
}
// Examples of using the function with different values
console.log(trailing_zeros_factorial(8)); // Output: 1 (factorial of 8 has one trailing zero)
console.log(trailing_zeros_factorial(9)); // Output: 1 (factorial of 9 has one trailing zero)
console.log(trailing_zeros_factorial(10)); // Output: 2 (factorial of 10 has two trailing zeros)
Output:
1 1 2
Live Demo:
See the Pen javascript-basic-exercise-112 by w3resource (@w3resource) on CodePen.
Flowchart:
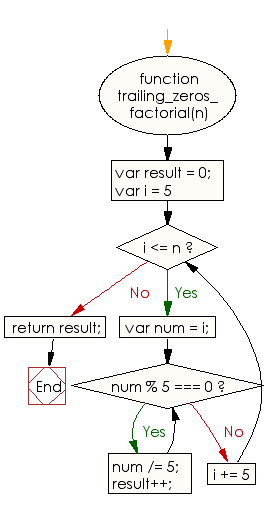
ES6 Version:
// ES6 code: Function to count trailing zeros in the factorial of a number
const trailing_zeros_factorial = (n) => {
let result = 0; // Initialize the count of trailing zeros to zero
for (let i = 5; i <= n; i += 5) { // Loop to calculate the factorial
let num = i; // Store the current number
while (num % 5 === 0) { // Check if the number is divisible by 5
num /= 5; // Divide the number by 5
result++; // Increment the count of trailing zeros
}
}
return result; // Return the total count of trailing zeros in the factorial of n
};
// Examples of using the function with different values
console.log(trailing_zeros_factorial(8)); // Output: 1 (factorial of 8 has one trailing zero)
console.log(trailing_zeros_factorial(9)); // Output: 1 (factorial of 9 has one trailing zero)
console.log(trailing_zeros_factorial(10)); // Output: 2 (factorial of 10 has two trailing zeros)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check a number from three given numbers where two numbers are equal, find the third one.
Next: JavaScript program to calculate the sum n + n/2 + n/4 + n/8 + .... where n is a positive integer and all divisions are integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics