JavaScript: Check a number from three given numbers where two numbers are equal, find the third one
JavaScript Basic: Exercise-111 with Solution
Find Unique Number Among Three
Write a JavaScript program to check a number from three given numbers where two numbers are equal. Find the third one.
Visual Presentation:
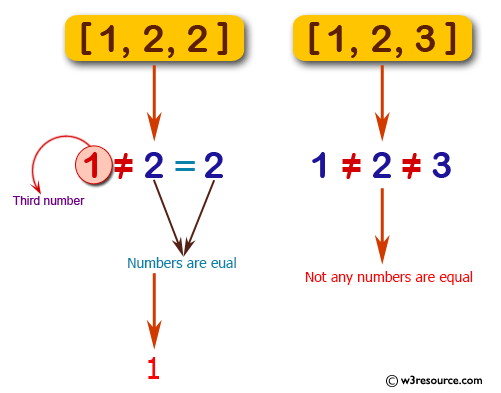
Sample Solution:
JavaScript Code:
// Function to find the third number that is different from the other two given numbers
function find_third_number(x, y, z) {
// Check if all three numbers are unequal
if ((x !== y) && (x !== z) && (y !== z)) {
return "Three numbers are unequal."; // Return a message if all three numbers are unequal
}
// Check if the first number is equal to the second number
if (x === y) {
return z; // Return the third number if the first and second numbers are equal
}
// Check if the first number is equal to the third number
if (x === z) {
return y; // Return the second number if the first and third numbers are equal
}
return x; // Return the first number (default case when second and third numbers are equal)
}
// Example usage of the function
console.log(find_third_number(1, 2, 2)); // Output: 1 (the third number different from the other two: 1)
console.log(find_third_number(1, 1, 2)); // Output: 2 (the third number different from the other two: 2)
console.log(find_third_number(1, 2, 3)); // Output: "Three numbers are unequal." (all three numbers are different)
Output:
1 2 Three numbers are unequal.
Live Demo:
See the Pen javascript-basic-exercise-111 by w3resource (@w3resource) on CodePen.
Flowchart:
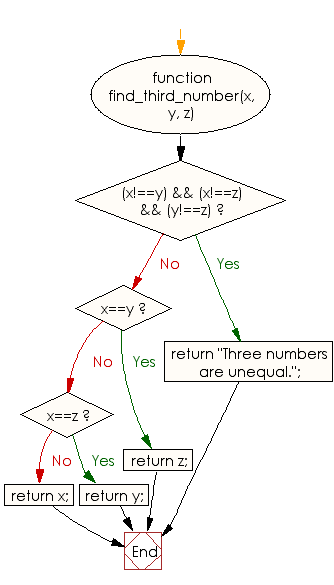
ES6 Version:
// Function to find the third number that is different from the other two given numbers
const find_third_number = (x, y, z) => {
if (x !== y && x !== z && y !== z) {
return "Three numbers are unequal."; // Return if all three numbers are different
}
if (x === y) {
return z; // Return 'z' if 'x' and 'y' are equal
}
if (x === z) {
return y; // Return 'y' if 'x' and 'z' are equal
}
return x; // Return 'x' if 'y' and 'z' are equal or all three are equal
};
// Example usage of the function
console.log(find_third_number(1, 2, 2)); // Output: 1 (x = 1, y = 2, z = 2, third number different from other two: 1)
console.log(find_third_number(1, 1, 2)); // Output: 2 (x = 1, y = 1, z = 2, third number different from other two: 2)
console.log(find_third_number(1, 2, 3)); // Output: "Three numbers are unequal." (All three numbers are different)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the number of even values in sequence before the first occurrence of a given number.
Next: JavaScript program to find the number of trailing zeros in the decimal representation of the factorial of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics