JavaScript: Find the number of even values in sequence before the first occurrence of a given number
JavaScript Basic: Exercise-110 with Solution
Count Evens Before First Occurrence of Number
Write a JavaScript program to find the number of even values in sequence before the first occurrence of a given number.
Sample sequence = [1,2,3,4,5,6,7,8]
Given number: 5
Output: 2
Sample Solution:
JavaScript Code:
// Function to count even numbers before the given number in the array
function find_numbers(arr_num, num) {
var result = 0; // Counter variable to store the count of even numbers
for (var i = 0; i < arr_num.length; i++) {
if (arr_num[i] % 2 === 0 && arr_num[i] !== num) { // Check if number is even and not equal to 'num'
result++; // Increment the counter for each even number
}
if (arr_num[i] === num) { // Check if the current number matches 'num'
return result; // Return the count of even numbers encountered so far
}
}
return -1; // Return -1 if 'num' is not found in the array
}
// Example usage of the function
console.log(find_numbers([1, 2, 3, 4, 5, 6, 7, 8], 5));
console.log(find_numbers([1, 3, 5, 6, 7, 8], 6));
Output:
2 0
Live Demo:
See the Pen javascript-basic-exercise-110 by w3resource (@w3resource) on CodePen.
Flowchart:
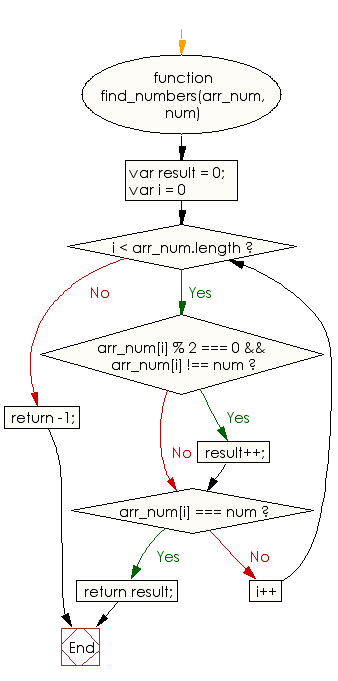
ES6 Version:
// Function to find numbers in an array that are even and not equal to a specific number
const find_numbers = (arr_num, num) => {
let result = 0; // Initialize the count of even numbers not equal to 'num'
for (let i = 0; i < arr_num.length; i++) {
if (arr_num[i] % 2 === 0 && arr_num[i] !== num) {
result++; // Increment count for even numbers not equal to 'num'
}
if (arr_num[i] === num) {
return result; // Return the count when 'num' is found in the array
}
}
return -1; // Return -1 if 'num' is not found in the array
};
// Example usage of the function
console.log(find_numbers([1, 2, 3, 4, 5, 6, 7, 8], 5)); // Output: 2 (even numbers not equal to 5: 2, 4)
console.log(find_numbers([1, 3, 5, 6, 7, 8], 6)); // Output: -1 (6 not found in the array)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that counts the number of even numbers in an array before the first occurrence of a specified target number.
- Write a JavaScript function that iterates through an array and stops counting evens once a given number is encountered.
- Write a JavaScript program that validates an array input and returns the count of even numbers preceding the first instance of a specified integer.
Go to:
PREV : Sort All Primes Between 1 and n.
NEXT : Find Unique Number Among Three.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.