JavaScript: Convert temperatures to and from celsius, fahrenheit
JavaScript Basic: Exercise-11 with Solution
Convert Temperatures Between Celsius and Fahrenheit
Write a JavaScript program to convert temperatures to and from celsius, fahrenheit.
JavaScript: Fahrenheit and Centigrade Temperatures:
Fahrenheit and centigrade are two temperature scales in use today. The Fahrenheit scale was developed by the German physicist Daniel Gabriel Fahrenheit . In the Fahrenheit scale, water freezes at 32 degrees and boils at 212 degrees.
The centigrade scale, which is also called the Celsius scale, was developed by Swedish astronomer Andres Celsius. In the centigrade scale, water freezes at 0 degrees and boils at 100 degrees. The centigrade to Fahrenheit conversion formula is:
C = (5/9) * (F - 32)
where F is the Fahrenheit temperature. You can also use this Web page to convert Fahrenheit temperatures to centigrade. Just enter a Fahrenheit temperature in the text box below, then click on the Convert button.
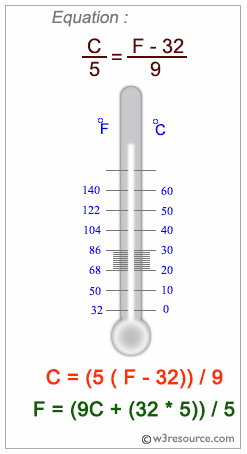
Sample Solution:
JavaScript Code:
// Define a function to convert Celsius to Fahrenheit
function cToF(celsius) {
// Store the input Celsius temperature in a variable
var cTemp = celsius;
// Calculate the equivalent Fahrenheit temperature
var cToFahr = cTemp * 9 / 5 + 32;
// Create a message string describing the conversion result
var message = cTemp + '\xB0C is ' + cToFahr + ' \xB0F.';
// Log the message to the console
console.log(message);
}
// Define a function to convert Fahrenheit to Celsius
function fToC(fahrenheit) {
// Store the input Fahrenheit temperature in a variable
var fTemp = fahrenheit;
// Calculate the equivalent Celsius temperature
var fToCel = (fTemp - 32) * 5 / 9;
// Create a message string describing the conversion result
var message = fTemp + '\xB0F is ' + fToCel + '\xB0C.';
// Log the message to the console
console.log(message);
}
// Call the cToF function with a Celsius temperature of 60
cToF(60);
// Call the fToC function with a Fahrenheit temperature of 45
fToC(45);
Output:
60°C is 140 °F. 45°F is 7.222222222222222°C.
Live Demo:
See the Pen JavaScript: Convert temperatures - basic-ex-11 by w3resource (@w3resource) on CodePen.
Explanation:
For an exact conversion (Fahrenheit to Celsius / Celsius to Fahrenheit) the following formulas can be applied :
Fahrenheit to Celsius : (°F − 32) ÷ 1.8 = °C
Celsius to Fahrenheit : (°C × 1.8) + 32 = °F
An approximate conversion between degrees Celsius and degrees Fahrenheit is as follows :
Fahrenheit to Celsius : Subtract 30 and halve the resulting number.
Celsius to Fahrenheit : Double the number and add 30.
Flowchart:
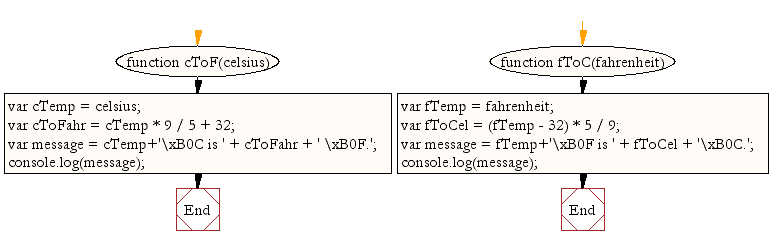
ES6 Version:
// Convert Celsius to Fahrenheit using arrow function
const cToF = (celsius) => {
const cTemp = celsius;
const cToFahr = cTemp * 9 / 5 + 32;
const message = `${cTemp}\xB0C is ${cToFahr} \xB0F.`;
console.log(message);
};
// Convert Fahrenheit to Celsius using arrow function
const fToC = (fahrenheit) => {
const fTemp = fahrenheit;
const fToCel = (fTemp - 32) * 5 / 9;
const message = `${fTemp}\xB0F is ${fToCel} \xB0C.`;
console.log(message);
};
// Example usage
cToF(60);
fToC(45);
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts an array of temperature values between Celsius and Fahrenheit.
- Write a JavaScript program that validates temperature input and performs conversions, alerting the user for non-numeric values.
- Write a JavaScript program that dynamically converts temperatures as the user types into an input field.
Go to:
PREV :Multiplication and Division (User Input).
NEXT : Get Current Website URL.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.