JavaScript: Sort an array of all prime numbers between 1 and a given integer
JavaScript Basic: Exercise-109 with Solution
Sort All Primes Between 1 and n
Write a JavaScript program to sort an array of all prime numbers between 1 and a given integer.
Sample Solution:
JavaScript Code:
// Function to sort prime numbers up to 'num'
function sort_prime(num) {
var prime_num1 = [], // Array to store prime numbers
prime_num2 = []; // Array to track prime status (true/false)
// Initialize prime_num2 array to true for all indices from 0 to num
for (var i = 0; i <= num; i++) {
prime_num2.push(true);
}
// Loop to find prime numbers using the Sieve of Eratosthenes algorithm
for (var i = 2; i <= num; i++) {
if (prime_num2[i]) {
prime_num1.push(i); // Push the current number to prime_num1 if it's a prime
// Mark multiples of the current number as non-prime in prime_num2
for (var j = 1; i * j <= num; j++) {
prime_num2[i * j] = false;
}
}
}
return prime_num1; // Return the array containing sorted prime numbers
}
// Example usage of the sort_prime function with different values
console.log(sort_prime(5)); // Output: [2, 3, 5]
console.log(sort_prime(11)); // Output: [2, 3, 5, 7, 11]
console.log(sort_prime(19)); // Output: [2, 3, 5, 7, 11, 13, 17, 19]
Output:
[2,3,5] [2,3,5,7,11] [2,3,5,7,11,13,17,19]
Live Demo:
See the Pen javascript-basic-exercise-109 by w3resource (@w3resource) on CodePen.
Flowchart:
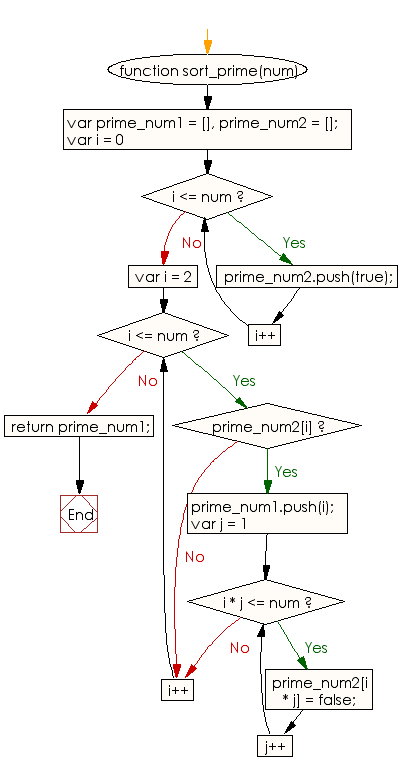
ES6 Version:
// Function to sort prime numbers up to a given number
const sort_prime = (num) => {
let prime_num1 = []; // Array to store prime numbers
let prime_num2 = []; // Array to hold flags indicating prime numbers
// Initializing flags in prime_num2 array
for (let i = 0; i <= num; i++) {
prime_num2.push(true);
}
// Finding prime numbers using the Sieve of Eratosthenes algorithm
for (let i = 2; i <= num; i++) {
if (prime_num2[i]) {
prime_num1.push(i); // Pushing the prime number to the prime_num1 array
// Marking multiples of the current prime number as non-prime in prime_num2
for (let j = 1; i * j <= num; j++) {
prime_num2[i * j] = false;
}
}
}
return prime_num1; // Returning the sorted array of prime numbers
};
// Example usage of the function
console.log(sort_prime(5)); // Output: [2, 3, 5] (prime numbers up to 5)
console.log(sort_prime(11)); // Output: [2, 3, 5, 7, 11] (prime numbers up to 11)
console.log(sort_prime(19)); // Output: [2, 3, 5, 7, 11, 13, 17, 19] (prime numbers up to 19)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates all prime numbers between 1 and n using the sieve of Eratosthenes, then sorts them in descending order.
- Write a JavaScript function that identifies prime numbers up to n and sorts the resulting array in ascending order.
- Write a JavaScript program that calculates primes up to a given n and sorts them based on the sum of their digits.
Go to:
PREV : Dot Product of Two 3D Vectors.
NEXT : Count Evens Before First Occurrence of Number.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.