JavaScript: Create the dot products of two given 3D vectors
JavaScript Basic: Exercise-108 with Solution
Dot Product of Two 3D Vectors
Write a JavaScript program to create the dot products of two given 3D vectors.
Note: The dot product is the sum of the products of the corresponding entries of the two sequences of numbers.
Sample Solution:
JavaScript Code:
// Function to calculate the dot product of two vectors in 3D space
function dot_product(vector1, vector2) {
var result = 0; // Initialize the variable to store the dot product result
// Loop through each component of the vectors and calculate the dot product
for (var i = 0; i < 3; i++) {
result += vector1[i] * vector2[i]; // Sum the products of corresponding components
}
return result; // Return the calculated dot product
}
// Examples of calculating dot products of given vectors
console.log(dot_product([1, 2, 3], [1, 2, 3])); // Output: 14 (1*1 + 2*2 + 3*3 = 14)
console.log(dot_product([2, 4, 6], [2, 4, 6])); // Output: 56 (2*2 + 4*4 + 6*6 = 56)
console.log(dot_product([1, 1, 1], [0, 1, -1])); // Output: 0 (1*0 + 1*1 + 1*(-1) = 0)
Output:
14 56 0
Live Demo:
See the Pen javascript-basic-exercise-109 by w3resource (@w3resource) on CodePen.
Flowchart:
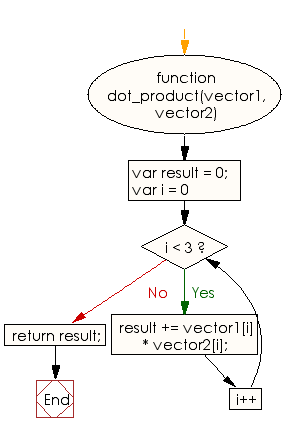
ES6 Version:
// Function to calculate the dot product of two 3-dimensional vectors
const dot_product = (vector1, vector2) => {
let result = 0; // Variable to store the dot product result
// Loop through the vectors and calculate the dot product
for (let i = 0; i < 3; i++) {
result += vector1[i] * vector2[i]; // Compute the dot product for each element pair
}
return result; // Return the final dot product value
};
// Example usage of the function
console.log(dot_product([1, 2, 3], [1, 2, 3])); // Output: 14 (1*1 + 2*2 + 3*3 = 14)
console.log(dot_product([2, 4, 6], [2, 4, 6])); // Output: 56 (2*2 + 4*4 + 6*6 = 56)
console.log(dot_product([1, 1, 1], [0, 1, -1])); // Output: 0 (1*0 + 1*1 + 1*(-1) = 0)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the dot product of two 3D vectors represented as arrays, including input validation.
- Write a JavaScript function that calculates the dot product by iterating over corresponding elements of two vectors.
- Write a JavaScript program that uses the reduce() method to compute the dot product of two given 3D vectors.
Go to:
PREV : Count Divisible Sorted Pairs in Array.
NEXT : Sort All Primes Between 1 and n.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.