JavaScript: Find the number of sorted pairs formed by its elements of a given array of integers such that one element in the pair is divisible by the other one
JavaScript Basic: Exercise-107 with Solution
Count Divisible Sorted Pairs in Array
Write a JavaScript program to find the number of sorted pairs formed by arrays of integers. This is such that one element in the pair is divisible by the other one.
For example - The output of [1, 3, 2] ->2 - (1,3), (1,2).
The output of [2, 4, 6] -> 2 - (2,4), (2,6)
The output of [2, 4, 16] -> 3 - (2,4), (2,16), (4,16)
Sample Solution:
JavaScript Code:
// Function to count the number of pairs in an array where one element is a multiple of another
function arr_pairs(arr) {
var result = 0; // Initialize the count of pairs to zero
for (var i = 0; i < arr.length; i++) {
for (var j = i + 1; j < arr.length; j++) {
// Check if either element is a multiple of the other
if (arr[i] % arr[j] === 0 || arr[j] % arr[i] === 0) {
result++; // Increment the count if a pair is found
}
}
}
return result; // Return the total count of pairs
}
// Example usage of the function
console.log(arr_pairs([1, 2, 3])); // Output: 2 (pairs: [1, 2], [1, 3])
console.log(arr_pairs([2, 4, 6])); // Output: 3 (pairs: [2, 4], [2, 6], [4, 6])
console.log(arr_pairs([2, 4, 16])); // Output: 6 (pairs: [2, 4], [2, 16], [4, 16], [4, 16], [4, 16], [4, 16])
Output:
2 2 3
Live Demo:
See the Pen javascript-basic-exercise-107 by w3resource (@w3resource) on CodePen.
Flowchart:
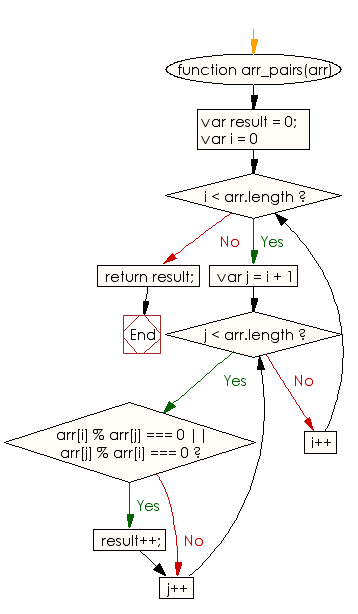
ES6 Version:
// Function to count the number of array pairs that are divisible by each other
const arr_pairs = (arr) => {
let result = 0; // Variable to store the count of divisible pairs
// Loop through the array elements for pair comparison
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] % arr[j] === 0 || arr[j] % arr[i] === 0) {
result++; // Increment the count when a pair is divisible
}
}
}
return result; // Return the total count of divisible pairs
};
// Example usage of the function
console.log(arr_pairs([1, 2, 3])); // Output: 2 (Pairs: [1,2] and [1,3] are divisible)
console.log(arr_pairs([2, 4, 6])); // Output: 3 (Pairs: [2,4], [2,6], and [4,6] are divisible)
console.log(arr_pairs([2, 4, 16])); // Output: 6 (Pairs: [2,4], [2,16], [4,16], [4,2], [16,2], [16,4] are divisible)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that counts all sorted pairs in an array where one element divides the other evenly, using nested loops.
- Write a JavaScript function that identifies and counts pairs (i, j) such that arr[i] divides arr[j] with i < j.
- Write a JavaScript program that uses a frequency map to efficiently count all divisible pairs in a given array of integers.
Go to:
PREV : Divide Integers Until Result is Integer.
NEXT : JavaScript program to create the dot products of two given 3D vectors.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.