JavaScript: Find the number of times to replace a given number with the sum of its digits until it convert to a single digit number
JavaScript Basic: Exercise-105 with Solution
Replace Number with Digit Sum Until Single Digit
Write a JavaScript program to find the number of times to replace a given number with the sum of its digits. This is until it converts to a single-digit number.
Visual Presentation:
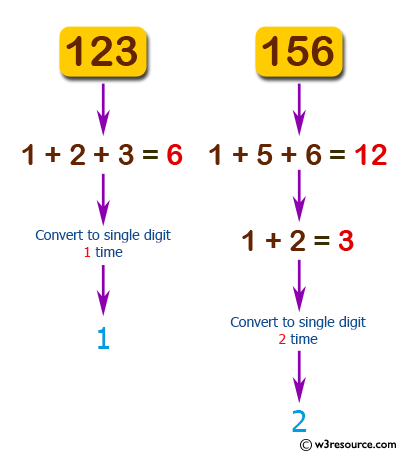
Sample Solution:
JavaScript Code:
// Function to calculate the sum of digits in a number
function digit_to_one(num) {
// Inner function to compute the sum of digits in a number
var digitSum = function(num) {
var digit_sum = 0; // Initialize the variable to store the sum of digits
while (num) { // Loop to extract digits and sum them
digit_sum += num % 10; // Add the last digit to the sum
num = Math.floor(num / 10); // Remove the last digit from the number
}
return digit_sum; // Return the sum of digits
};
var result = 0; // Initialize the counter for the number of steps
while (num >= 10) { // Loop until the number becomes a single digit
result += 1; // Increment the counter for each step
num = digitSum(num); // Get the sum of digits and assign it to the number
}
return result; // Return the count of steps required
}
// Example usage of the function
console.log(digit_to_one(123));
console.log(digit_to_one(156));
Output:
1 2
Live Demo:
See the Pen javascript-basic-exercise-105 by w3resource (@w3resource) on CodePen.
Flowchart:
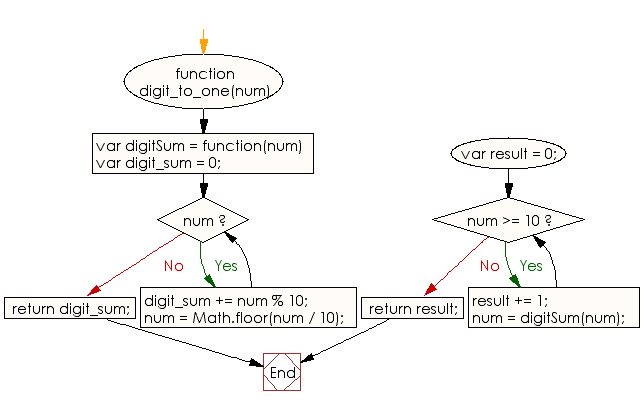
ES6 Version:
// Function to calculate the sum of digits in a number
const digitSum = (num) => {
let digit_sum = 0; // Initialize sum of digits variable
while (num) { // Loop to calculate the sum of digits
digit_sum += num % 10; // Add the last digit to the sum
num = Math.floor(num / 10); // Remove the last digit from the number
}
return digit_sum; // Return the sum of digits
};
// Function to convert a number to one digit by finding the sum of its digits until it becomes a single digit
const digit_to_one = (num) => {
let result = 0; // Initialize count of steps to convert number to a single digit
while (num >= 10) { // Loop until the number becomes a single digit
result += 1; // Increment the step count
num = digitSum(num); // Calculate the sum of digits and assign it to the number
}
return result; // Return the count of steps taken
};
// Example usage of the function
console.log(digit_to_one(123));
console.log(digit_to_one(156));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that repeatedly replaces a number with the sum of its digits until the result is a single digit, and counts the iterations.
- Write a JavaScript function that applies the digit sum operation recursively and returns both the final single-digit result and the iteration count.
- Write a JavaScript program that simulates the process of digit summing, stopping when a single-digit number is reached, and handles large numbers efficiently.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find two elements of the array such that their absolute difference is not greater than a given integer but is as close to the said integer.
Next: JavaScript program to divide an integer by another integer as long as the result is an integer and return the result.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.