JavaScript: Find two elements of the array such that their absolute difference is not greater than a given integer but is as close to the said integer
JavaScript Basic: Exercise-104 with Solution
Find Pair with Closest Absolute Difference
Write a JavaScript program to find two elements of an array such that their absolute difference is not larger than a given integer. However, it is as close as possible to the integer.
Sample Solution:
JavaScript Code:
// Function to find the maximum absolute difference between elements of an array that is less than or equal to a given number
function different_values(ara, n) {
var max_val = -1; // Initializing the maximum value as -1
for (var i = 0; i < ara.length; i++) { // Looping through the array elements
for (var j = i + 1; j < ara.length; j++) { // Nested loop for comparisons with subsequent elements
var x = Math.abs(ara[i] - ara[j]); // Calculating the absolute difference between elements
if (x <= n) { // Checking if the absolute difference is less than or equal to 'n'
max_val = Math.max(max_val, x); // Updating the maximum value if the condition is met
}
}
}
return max_val; // Returning the maximum value found
}
// Examples of using the function with different arrays and values of 'n'
console.log(different_values([12, 10, 33, 34], 10)); // Example usage
console.log(different_values([12, 10, 33, 34], 24)); // Example usage
console.log(different_values([12, 10, 33, 44], 40)); // Example usage
Output:
2 24 34
Live Demo:
See the Pen javascript-basic-exercise-104 by w3resource (@w3resource) on CodePen.
Flowchart:
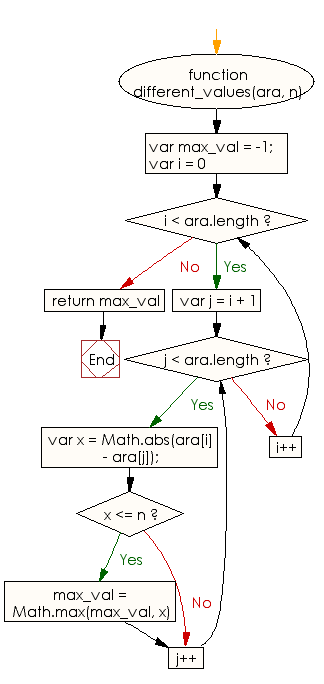
ES6 Version:
// Function to find the maximum difference between two array elements that are less than or equal to a given number
const different_values = (ara, n) => {
let max_val = -1; // Initialize maximum difference variable to track the result
for (let i = 0; i < ara.length; i++) { // Loop through the array elements
for (let j = i + 1; j < ara.length; j++) { // Loop through subsequent elements to compare differences
let x = Math.abs(ara[i] - ara[j]); // Calculate the absolute difference between array elements
if (x <= n) { // Check if the difference is less than or equal to the given number
max_val = Math.max(max_val, x); // Update the maximum difference if the current difference is greater
}
}
}
return max_val; // Return the maximum difference found
}
// Example usage of the function
console.log(different_values([12, 10, 33, 34], 10));
console.log(different_values([12, 10, 33, 34], 24));
console.log(different_values([12, 10, 33, 44], 40));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds two elements in an array whose absolute difference is as close as possible to a given target without exceeding it.
- Write a JavaScript function that sorts an array and then identifies the pair with the absolute difference nearest to a specified threshold.
- Write a JavaScript program that iterates through all pairs in a sorted array to return the one with the minimal difference compared to a given value.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the maximum number from a given positive integer by deleting exactly one digit of the given number.
Next: JavaScript program to find the number of times to replace a given number with the sum of its digits until it convert to a single digit number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.