JavaScript: Find the maximum number from a given positive integer by deleting exactly one digit of the given number
JavaScript Basic: Exercise-103 with Solution
Max Integer by Removing One Digit
Write a JavaScript program to find the maximum number of a given positive integer by deleting exactly one digit of the given number.
Visual Presentation:
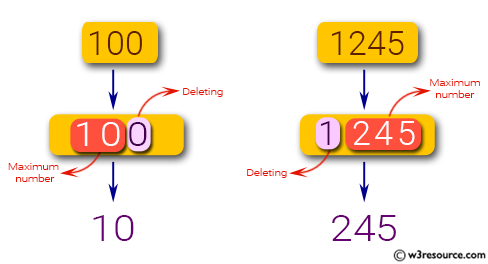
Sample Solution:
JavaScript Code:
// Function to delete a digit from a given number and return the maximum resulting number
function digit_delete(num) {
var result = 0, // Variable to store the maximum resulting number
num_digits = []; // Array to store individual digits of the number
// Extract digits of the number and store them in an array
while (num) {
num_digits.push(num % 10);
num = Math.floor(num / 10);
}
// Iterate through each digit and generate numbers after deleting each digit
for (var index_num = 0; index_num < num_digits.length; index_num++) {
var n = 0; // Temporary variable to store the generated number
// Create a new number without the current indexed digit
for (var i = num_digits.length - 1; i >= 0; i--) {
if (i !== index_num) {
n = n * 10 + num_digits[i];
}
}
// Store the maximum number obtained after deleting each digit
result = Math.max(n, result);
}
return result; // Return the maximum resulting number
}
// Test cases
console.log(digit_delete(100)); // Example usage
console.log(digit_delete(10)); // Example usage
console.log(digit_delete(1245)); // Example usage
Output:
10 1 245
Live Demo:
See the Pen javascript-basic-exercise-103 by w3resource (@w3resource) on CodePen.
Flowchart:
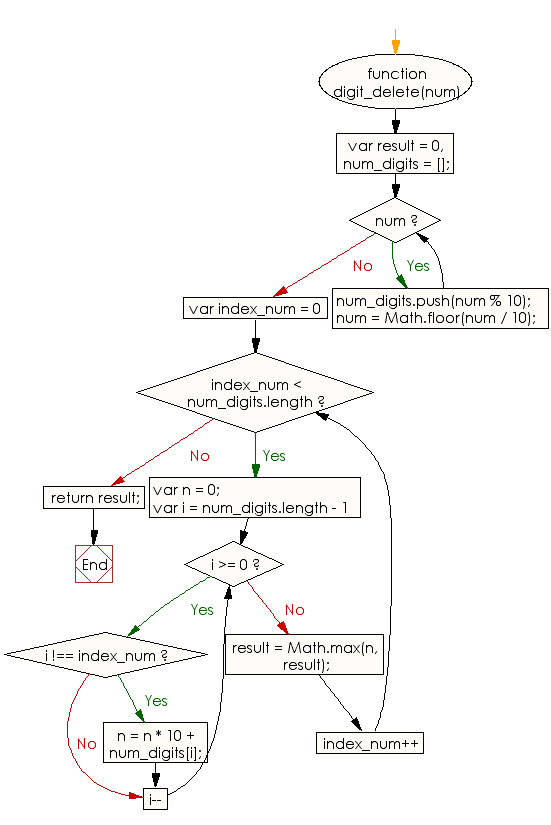
ES6 Version:
// Function to delete a digit from a number and return the highest possible number after deletion
const digit_delete = (num) => {
let result = 0; // Initialize the result variable
let num_digits = []; // Array to store digits of the number
// Extract digits from the number and store them in an array
while (num) {
num_digits.push(num % 10);
num = Math.floor(num / 10);
}
// Loop through each digit to delete and find the maximum number after deletion
for (let index_num = 0; index_num < num_digits.length; index_num++) {
let n = 0; // Initialize a temporary variable for number formation
for (let i = num_digits.length - 1; i >= 0; i--) {
if (i !== index_num) {
n = n * 10 + num_digits[i]; // Form a number excluding the indexed digit
}
}
result = Math.max(n, result); // Update the result with the maximum number obtained
}
return result; // Return the highest possible number after deletion
}
console.log(digit_delete(100)); // Example usage
console.log(digit_delete(10)); // Example usage
console.log(digit_delete(1245)); // Example usage
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the maximum possible integer by removing exactly one digit from a given number, considering edge cases with duplicate digits.
- Write a JavaScript function that iterates over the digits of a number to determine which digit’s removal yields the highest result.
- Write a JavaScript program that tests each possible one-digit removal from an integer and returns the maximum remaining value.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the number of inversions of a given array of integers.
Next: JavaScript program to find two elements of the array such that their absolute difference is not greater than a given integer but is as close to the said integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.