JavaScript: Check whether a given string contains only Latin letters and no two uppercase and no two lowercase letters are in adjacent positions
JavaScript Basic: Exercise-101 with Solution
Check Latin Letters with No Adjacent Upper/Lower Case
Write a JavaScript program to check whether a given string contains only Latin letters and no two uppercase and no two lowercase letters are in adjacent positions.
Visual Presentation:
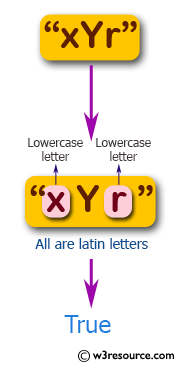
Sample Solution:
JavaScript Code:
// Function to test a string
function test_string(input_str) {
// Function to check if symbol is lowercase
var is_lower_case = function(symbol) {
if ('a' <= symbol && symbol <= 'z') {
return true;
}
return false;
}
// Function to check if symbol is uppercase
var is_upper_case = function(symbol) {
if ('A' <= symbol && symbol <= 'Z') {
return true;
}
return false;
}
// Check if the first character is lowercase or uppercase
var is_first_char_lower = is_lower_case(input_str[0]),
is_first_char_upper = is_upper_case(input_str[0]);
// If the first character is neither lowercase nor uppercase, return false
if (!(is_first_char_lower || is_first_char_upper)) {
return false;
}
// Iterate through the string
for (var i = 1; i < input_str.length; i++) {
// Check odd positions
if (i % 2) {
// Check if the character's case differs from the first character's case
if (is_lower_case(input_str[i]) === is_first_char_lower ||
is_upper_case(input_str[i]) === is_first_char_upper) {
return false; // Return false if cases are the same
}
} else {
// Check if the character's case matches the first character's case
if (is_lower_case(input_str[i]) !== is_first_char_lower ||
is_upper_case(input_str[i]) !== is_first_char_upper) {
return false; // Return false if cases do not match
}
}
}
return true; // Return true if conditions are met for the entire string
}
console.log(test_string('xYr')); // Example usage
console.log(test_string('XXyx')); // Example usage
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-101 by w3resource (@w3resource) on CodePen.
Flowchart:
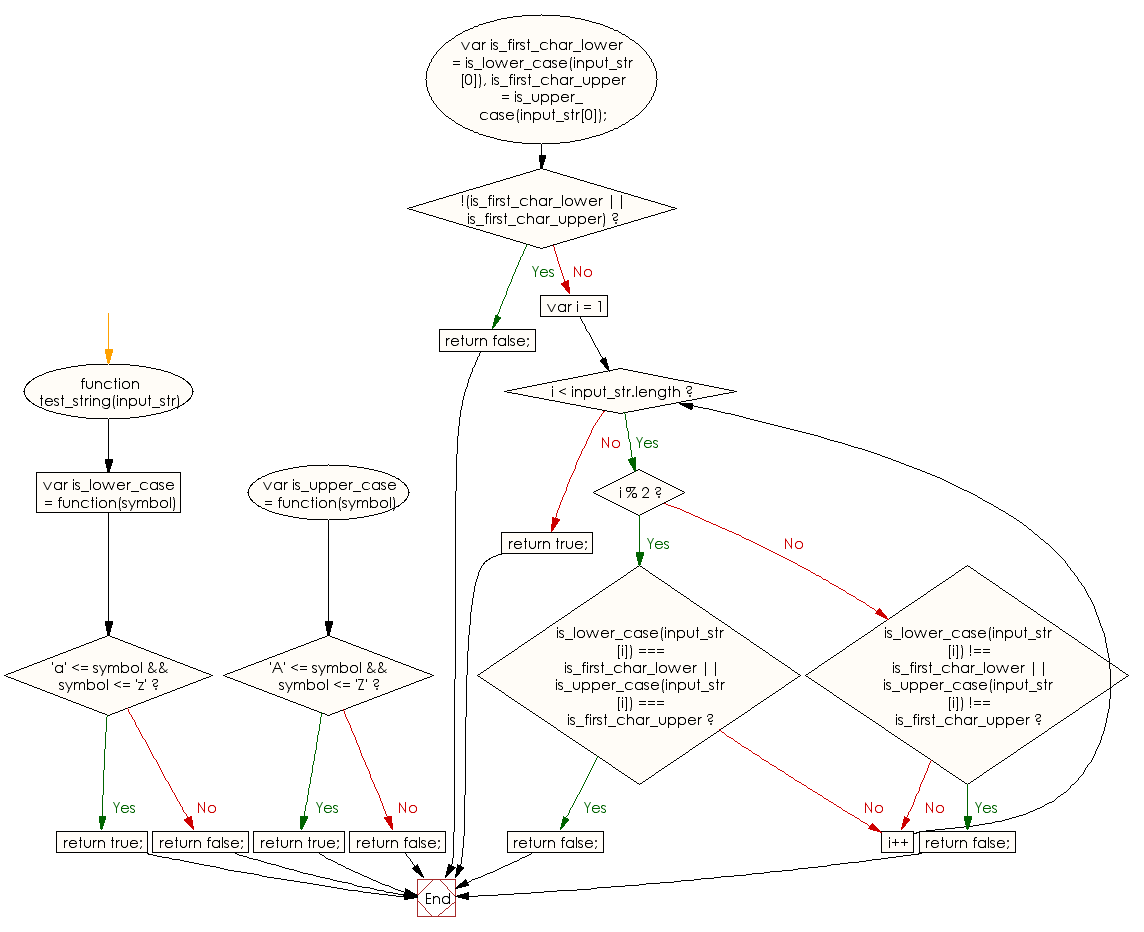
ES6 Version:
// Function to test a string based on specific conditions
const test_string = (input_str) => {
// Function to check if a symbol is a lowercase letter
const is_lower_case = (symbol) => {
if ('a' <= symbol && symbol <= 'z') {
return true;
}
return false;
}
// Function to check if a symbol is an uppercase letter
const is_upper_case = (symbol) => {
if ('A' <= symbol && symbol <= 'Z') {
return true;
}
return false;
}
// Determine if the first character is lowercase or uppercase
const is_first_char_lower = is_lower_case(input_str[0]);
const is_first_char_upper = is_upper_case(input_str[0]);
// If the first character is neither lowercase nor uppercase, return false
if (!(is_first_char_lower || is_first_char_upper)) {
return false;
}
// Iterate through the string and check conditions based on index and character case
for (let i = 1; i < input_str.length; i++) {
if (i % 2) {
if ((is_lower_case(input_str[i]) === is_first_char_lower) ||
(is_upper_case(input_str[i]) === is_first_char_upper)) {
return false;
}
} else {
if ((is_lower_case(input_str[i]) !== is_first_char_lower) ||
(is_upper_case(input_str[i]) !== is_first_char_upper)) {
return false;
}
}
}
// If all conditions are met, return true
return true;
}
console.log(test_string('xYr')); // Example usage
console.log(test_string('XXyx')); // Example usage
For more Practice: Solve these Related Problems:
- Write a JavaScript program that verifies a string contains only Latin letters and that no two adjacent letters share the same case using regular expressions.
- Write a JavaScript function that checks if a string follows an alternating pattern of uppercase and lowercase letters for all Latin characters.
- Write a JavaScript program that scans a string and returns true only if every letter alternates in case and no two consecutive letters are both uppercase or both lowercase.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if there is at least one element which occurs in two given sorted arrays of integers.
Next: JavaScript program to find the number of inversions of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.