JavaScript: Swap the case of each character of a string, upper case to lower and vice versa
JavaScript Array: Exercise-9 with Solution
Swap Case in String
Write a JavaScript program that accepts a string as input and swaps the case of each character. For example if you input 'The Quick Brown Fox' the output should be 'tHE qUICK bROWN fOX'.
Visual Presentation:
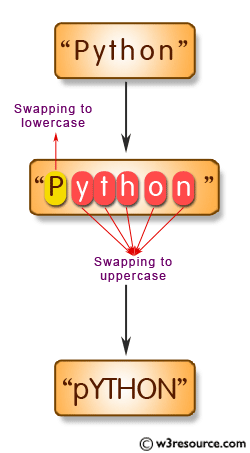
Sample Solution:
JavaScript Code:
// Declare and initialize the input string
var str = 'c';
// Define constants for uppercase and lowercase letters
var UPPER = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
var LOWER = 'abcdefghijklmnopqrstuvwxyz';
// Initialize an array to store the result
var result = [];
// Iterate through each character in the input string
for (var x = 0; x < str.length; x++) {
// Check if the current character is an uppercase letter
if (UPPER.indexOf(str[x]) !== -1) {
// Convert the uppercase letter to lowercase and add it to the result array
result.push(str[x].toLowerCase());
}
// Check if the current character is a lowercase letter
else if (LOWER.indexOf(str[x]) !== -1) {
// Convert the lowercase letter to uppercase and add it to the result array
result.push(str[x].toUpperCase());
}
// If the current character is neither uppercase nor lowercase, add it to the result array as is
else {
result.push(str[x]);
}
}
// Output the joined result array as a string
console.log(result.join(''));
Output:
C
Flowchart:
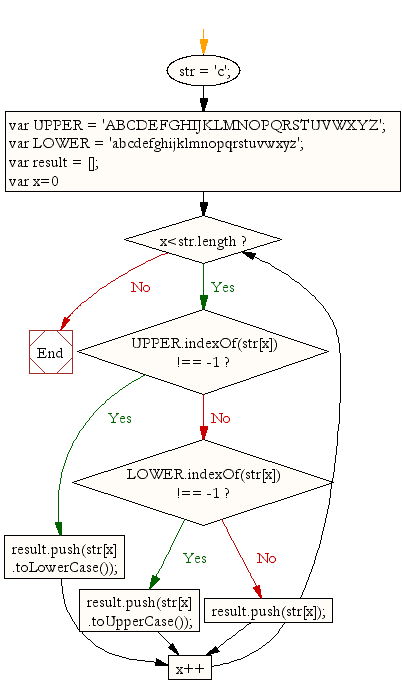
ES6 Version:
// Declare and initialize the input string
const str = 'c';
// Define constants for uppercase and lowercase letters
const UPPER = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
const LOWER = 'abcdefghijklmnopqrstuvwxyz';
// Initialize an array to store the result
const result = [];
// Iterate through each character in the input string
for (let x = 0; x < str.length; x++) {
// Check if the current character is an uppercase letter
if (UPPER.indexOf(str[x]) !== -1) {
// Convert the uppercase letter to lowercase and add it to the result array
result.push(str[x].toLowerCase());
}
// Check if the current character is a lowercase letter
else if (LOWER.indexOf(str[x]) !== -1) {
// Convert the lowercase letter to uppercase and add it to the result array
result.push(str[x].toUpperCase());
}
// If the current character is neither uppercase nor lowercase, add it to the result array as is
else {
result.push(str[x]);
}
}
// Output the joined result array as a string
console.log(result.join(''));
Live Demo:
See the Pen JavaScript - Swap the case of each character of a string, upper case to lower and vice versa- array-ex- 9 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that swaps the case of every character in a string using a loop and character code conversion.
- Write a JavaScript function that employs regular expressions to change lowercase letters to uppercase and vice versa.
- Write a JavaScript function that leaves non-alphabetical characters unchanged while swapping the case of letters.
- Write a JavaScript function that splits a string into an array, swaps each character’s case, and then rejoins the array into a string.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to find the most frequent item of an array.
Next: Write a JavaScript program which prints the elements of the following array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics