JavaScript: Find the most frequent item of an array
JavaScript Array: Exercise-8 with Solution
Most Frequent Array Item
Write a JavaScript program to find the most frequent item in an array.
Sample array: var arr1=[3, 'a', 'a', 'a', 2, 3, 'a', 3, 'a', 2, 4, 9, 3];
Sample Output: a ( 5 times )
Visual Presentation:
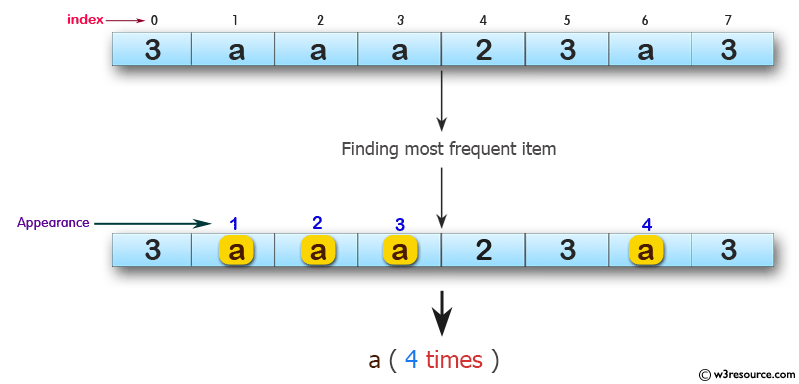
Sample Solution:
JavaScript Code:
// Declare and initialize the original array
var arr1 = [3, 'a', 'a', 'a', 2, 3, 'a', 3, 'a', 2, 4, 9, 3];
// Initialize variables to track the most frequent item, its frequency, and the current item's frequency
var mf = 1;
var m = 0;
var item;
// Iterate through the array to find the most frequent item
for (var i = 0; i < arr1.length; i++) {
// Nested loop to compare the current item with others in the array
for (var j = i; j < arr1.length; j++) {
// Check if the current item matches with another item in the array
if (arr1[i] == arr1[j])
m++;
// Update the most frequent item and its frequency if the current item's frequency is higher
if (mf < m) {
mf = m;
item = arr1[i];
}
}
// Reset the current item's frequency for the next iteration
m = 0;
}
// Output the most frequent item and its frequency
console.log(item + " ( " + mf + " times ) ");
Output:
a ( 5 times )
Flowchart:
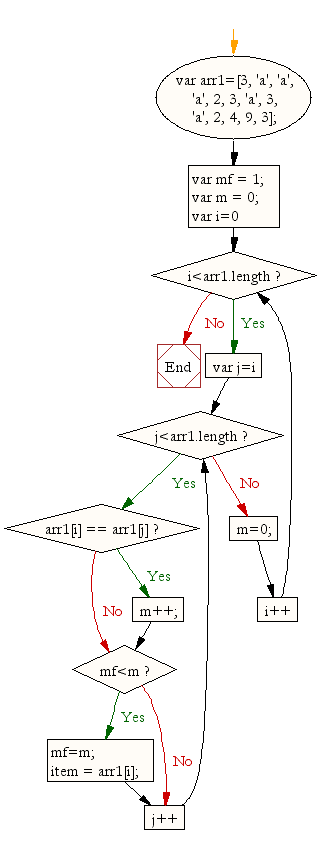
ES6 Version:
// Declare and initialize the original array
const arr1 = [3, 'a', 'a', 'a', 2, 3, 'a', 3, 'a', 2, 4, 9, 3];
// Initialize variables to track the most frequent item, its frequency, and the current item's frequency
let mf = 1;
let m = 0;
let item;
// Iterate through the array to find the most frequent item
for (let i = 0; i < arr1.length; i++) {
// Nested loop to compare the current item with others in the array
for (let j = i; j < arr1.length; j++) {
// Check if the current item matches with another item in the array
if (arr1[i] == arr1[j])
m++;
// Update the most frequent item and its frequency if the current item's frequency is higher
if (mf < m) {
mf = m;
item = arr1[i];
}
}
// Reset the current item's frequency for the next iteration
m = 0;
}
// Output the most frequent item and its frequency
console.log(item + " ( " + mf + " times ) ");
Live Demo:
See the Pen JavaScript - Find the most frequent item of an array- array-ex- 8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the most frequent item in an array and returns both the item and its occurrence count.
- Write a JavaScript function that handles arrays with mixed data types when determining the frequency of items.
- Write a JavaScript function that uses a hash map to compute the frequency of items and returns the one with the highest count.
- Write a JavaScript function that resolves ties by returning the item that appears first in the array when frequencies are equal.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to sort the items of an array.
Next: Write a JavaScript program which accept a string as input and swap the case of each character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.