JavaScript: Insert dashes (-) between each two even digits of a specific number
JavaScript Array: Exercise-6 with Solution
Insert Dashes Between Evens
Write a JavaScript program that accepts a number as input and inserts dashes (-) between each even number. For example if you accept 025468 the output should be 0-254-6-8.
Sample Solution:
JavaScript Code:
// Prompt the user for input and store it in the 'num' variable
var num = window.prompt();
// Convert the number to a string and store it in the 'str' variable
var str = num.toString();
// Initialize an array 'result' with the first character of the string
var result = [str[0]];
// Iterate through the characters of the string starting from index 1
for (var x = 1; x < str.length; x++) {
// Check if the previous and current characters are both even digits
if (str[x - 1] % 2 === 0 && str[x] % 2 === 0) {
// If both are even, push a '-' and the current character to the 'result' array
result.push('-', str[x]);
} else {
// If not both even, push the current character to the 'result' array
result.push(str[x]);
}
}
// Output the joined 'result' array as a string
console.log(result.join(''));
Output:
0-254-6-8
Flowchart:
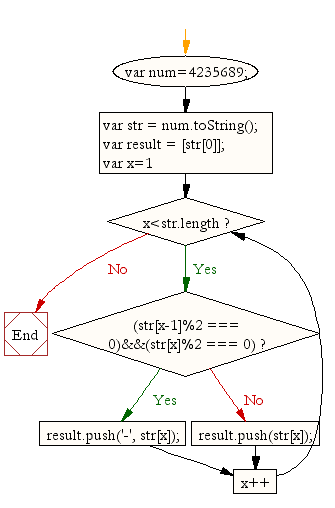
ES6 Version:
// Prompt the user for input and store it in the 'num' variable
const num = window.prompt();
// Convert the number to a string and store it in the 'str' variable
const str = num.toString();
// Initialize an array 'result' with the first character of the string
const result = [str[0]];
// Iterate through the characters of the string starting from index 1
for (let x = 1; x < str.length; x++) {
// Check if the previous and current characters are both even digits
if (str[x - 1] % 2 === 0 && str[x] % 2 === 0) {
// If both are even, push a '-' and the current character to the 'result' array
result.push('-', str[x]);
} else {
// If not both even, push the current character to the 'result' array
result.push(str[x]);
}
}
// Output the joined 'result' array as a string
console.log(result.join(''));
Live Demo:
See the Pen JavaScript - Insert dashes (-) between each two even digits of a specific number- array-ex-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that accepts a number as a string and inserts dashes between every two consecutive even digits.
- Write a JavaScript function that processes a numeric string and correctly handles leading zeros when inserting dashes.
- Write a JavaScript function that validates the input and returns the original string if no consecutive even digits exist.
- Write a JavaScript function that implements dash insertion without converting the string into an array.
Improve this sample solution and post your code through Disqus.
Previous: Write a simple JavaScript program to join all elements of the following array into a string.
Next: Write a JavaScript program to sort the items of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.