JavaScript: Number of arrays inside an array
JavaScript Array: Exercise-53 with Solution
Write a JavaScript program to count the number of arrays inside a given array.
Test Data:
([2,8,[6],3,3,5,3,4,[5,4]]) -> 2
([2,8,[6,3,3],[4],5,[3,4,[5,4]]]) -> 3
Sample Solution:
JavaScript Code-1:
// Function to count the number of arrays inside an array
function test(arr_nums){
// Use filter to create an array containing only the arrays from the input array
return arr_nums.filter(n => Array.isArray(n)).length;
}
// Test the function with an array containing nested arrays
arr_nums = [2, 8, [6], 3, 3, 5, 3, 4, [5, 4]];
console.log("Number of arrays inside the said array: " + test(arr_nums));
// Test the function with another array containing nested arrays
arr_nums = [2, 8, [6, 3, 3], [4], 5, [3, 4, [5, 4]]];
console.log("Number of arrays inside the said array: " + test(arr_nums));
Output:
Number of arrays inside the said array: 2 Number of arrays inside the said array: 3
Flowchart :
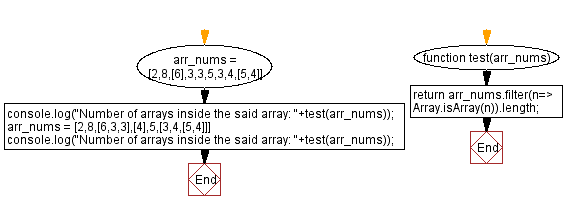
Live Demo :
See the Pen javascript-array-exercise-53 by w3resource (@w3resource) on CodePen.
JavaScript Code-2:
// Function to count the number of non-empty arrays inside an array
function test(arr_nums){
// Use filter to create an array containing only non-empty arrays from the input array
return arr_nums.filter(n => n.length).length;
}
// Test the function with an array containing nested arrays
arr_nums = [2, 8, [6], 3, 3, 5, 3, 4, [5, 4]];
console.log("Number of non-empty arrays inside the said array: " + test(arr_nums));
// Test the function with another array containing nested arrays
arr_nums = [2, 8, [6, 3, 3], [4], 5, [3, 4, [5, 4]]];
console.log("Number of non-empty arrays inside the said array: " + test(arr_nums));
Output:
Number of arrays inside the said array: 2 Number of arrays inside the said array: 3
Flowchart :
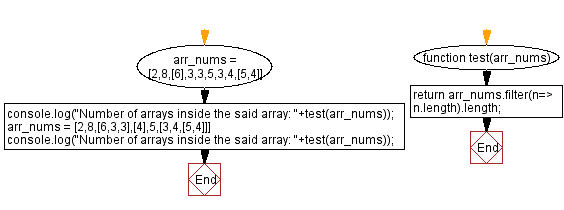
Live Demo :
See the Pen javascript-array-exercise-53-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous:Check if an array is a factor chain.
Next: Javascript Date Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-array-exercise-53.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics