JavaScript: Sum all numbers of an array of mixed data types
JavaScript Array: Exercise-50 with Solution
Sum Numbers in Mixed Array
Write a JavaScript program that takes an array with mixed data type and calculates the sum of all numbers.
Test Data:
([2, "11", 3, "a2", false, 5, 7, 1]) -> 18
([2, 3, 0, 5, 7, 8, true, false]) -> 25
Sample Solution:
JavaScript Code :
// Function to calculate the sum of all numbers in a mixed array
function test(arr_mix) {
// Initialize a variable 'total' to store the sum
var total = 0;
// Iterate through the elements of the array
for (var i = 0; i <= arr_mix.length; i++) {
// Check if the current element is a number
if (typeof arr_mix[i] === "number")
// Add the number to the 'total'
total = total + arr_mix[i];
}
// Return the calculated total
return total;
}
// Test the function with an array containing mixed data types
arr_mix = [2, "11", 3, "a2", false, 5, 7, 1];
console.log("Original array: " + arr_mix);
console.log("Sum all numbers of the said array: " + test(arr_mix));
// Test the function with another array containing mixed data types
arr_mix = [2, 3, 0, 5, 7, 8, true, false];
console.log("Original array: " + arr_mix);
console.log("Sum all numbers of the said array: " + test(arr_mix));
Output:
Original array: 2,11,3,a2,false,5,7,1 Sum all numbers of the said array: 18 Original array: 2,3,0,5,7,8,true,false Sum all numbers of the said array: 25
Flowchart :
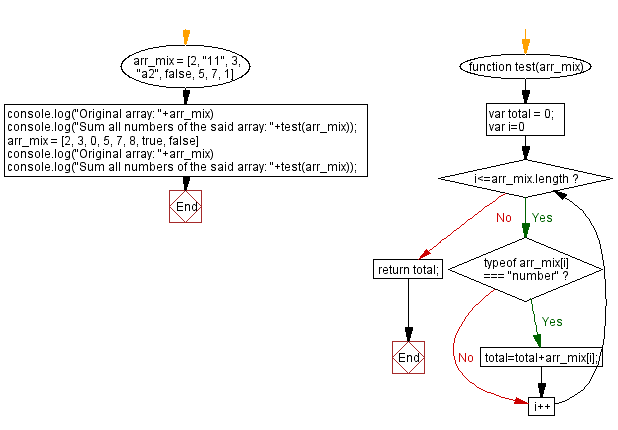
Live Demo :
See the Pen javascript-array-exercise-50 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous:Third smallest number of an array of numbers.
Next: Check if an array is a factor chain.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics