JavaScript: Third smallest number of an array of numbers
JavaScript Array: Exercise-49 with Solution
Find Third Smallest Number
Write a JavaScript program that takes an array of numbers and returns the third smallest number.
Test Data:
(2,3,5,7,1) -> 3
(2,3,0,5,7,8,-2,-4) -> 0
Sample Solution:
JavaScript Code :
// Function to find the third smallest number in an array
function test(arr_nums) {
// Sort the array in descending order using the compare function (y - x)
// and return the third element (index 2) from the sorted array
return arr_nums.sort((x, y) => y - x)[arr_nums.length - 3];
}
// Test the function with an array of numbers
nums = [2, 3, 5, 7, 1];
console.log("Original array of numbers: " + nums);
console.log("Third smallest number of the said array of numbers: " + test(nums));
// Test the function with another array of numbers
nums = [2, 3, 0, 5, 7, 8, -2, -4];
console.log("Original array of numbers: " + nums);
console.log("Third smallest number of the said array of numbers: " + test(nums));
Output:
Original array of numbers: 2,3,5,7,1 Third smallest number of the said array of numbers: 3 Original array of numbers: 2,3,0,5,7,8,-2,-4 Third smallest number of the said array of numbers: 0
Flowchart :
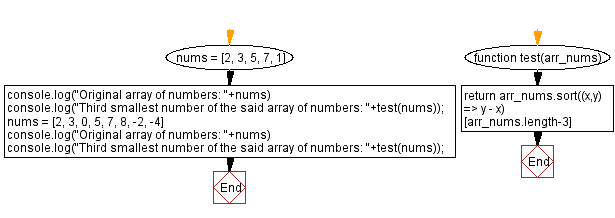
Live Demo :
See the Pen javascript-array-exercise-49 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts an array and returns the third smallest element.
- Write a JavaScript function that uses a min-heap to extract the three smallest numbers and returns the third one.
- Write a JavaScript function that iterates through an array to track the three smallest values without full sorting.
- Write a JavaScript function that handles duplicate values and returns the third unique smallest number.
Improve this sample solution and post your code through Disqus.
Previous:Check every numbers are prime or not in an array.
.
Next: Sum all numbers of an array of mixed data types.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.