JavaScript: Ungrouping the elements in an array produced by zip
JavaScript Array: Exercise-43 with Solution
Unzip Arrays
Write a JavaScript function to create an array of arrays, ungrouping the elements in an array produced by zip.
Use Math.max(), Function.prototype.apply() to get the longest subarray in the array, Array.prototype.map() to make each element an array.
Use Array.prototype.reduce() and Array.prototype.forEach() to map grouped values to individual arrays.
Sample Solution:
JavaScript Code :
// Source: https://bit.ly/3hEZdCl
// Function to unzip an array of arrays
const unzip = arr =>
// Reduce the array, merging elements by index
arr.reduce(
(acc, val) => (val.forEach((v, i) => acc[i].push(v)), acc),
// Initialize an array with empty arrays, one for each index
Array.from({
length: Math.max(...arr.map(x => x.length))
}).map(x => [])
);
// Output the result of unzipping the given arrays
console.log(unzip([['a', 1, true], ['b', 2, false]]));
console.log(unzip([['a', 1, true], ['b', 2]]));
Output:
[["a","b"],[1,2],[true,false]] [["a","b"],[1,2],[true]]
Flowchart :
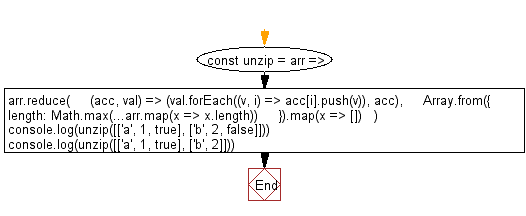
Live Demo :
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that takes a zipped array (an array of arrays) and returns separate arrays for each position.
- Write a JavaScript function that unzips a nested array by grouping elements from each subarray based on their index.
- Write a JavaScript function that validates that all subarrays have the same length before performing the unzip operation.
- Write a JavaScript function that handles uneven subarray lengths by unzipping only up to the length of the shortest subarray.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find the unique elements from two arrays.
Next: Write a JavaScript function to create an object from an array, using the specified key and excluding it from each value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.