JavaScript: Find the unique elements from two arrays
JavaScript Array: Exercise-42 with Solution
Unique Elements from Two Arrays
Write a JavaScript function to find unique elements in two arrays.
Test Data :
console.log(difference([1, 2, 3], [100, 2, 1, 10]));
["1", "2", "3", "10", "100"]
console.log(difference([1, 2, 3, 4, 5], [1, [2], [3, [[4]]],[5,6]]));
["1", "2", "3", "4", "5", "6"]
console.log(difference([1, 2, 3], [100, 2, 1, 10]));
["1", "2", "3", "10", "100"]
Visual Presentation:
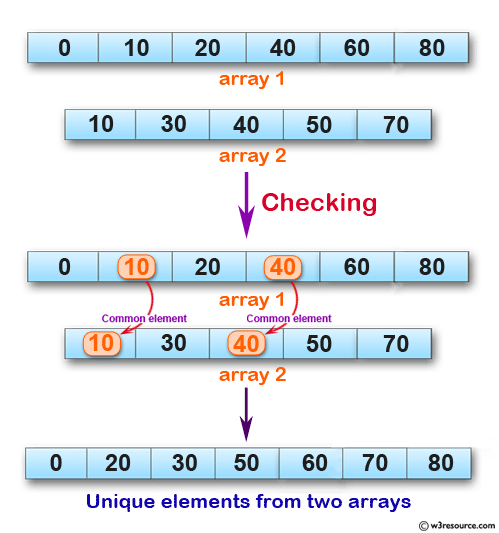
Sample Solution:
JavaScript Code :
Output:
["1","2","3","10","100"] ["1","2","3","4","5","6"] ["1","2","3","10","100"]
Flowchart :
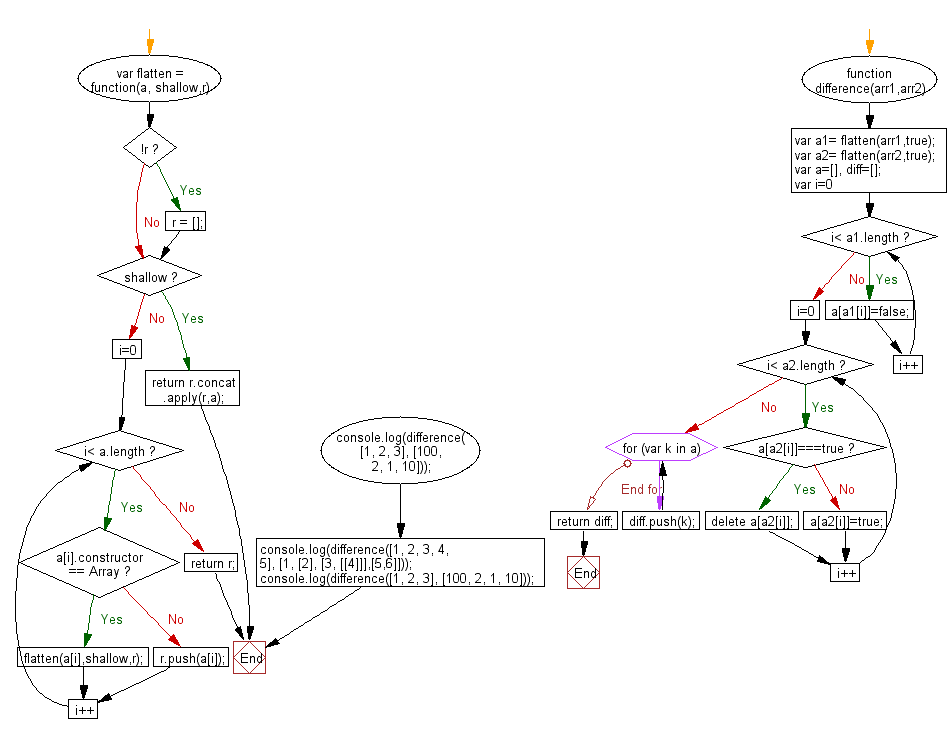
ES6 Version:
Live Demo :
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds elements unique to each of two arrays and returns their union without duplicates.
- Write a JavaScript function that uses Set operations to determine unique elements present in either array.
- Write a JavaScript function that manually compares two arrays and extracts elements that are not common to both.
- Write a JavaScript function that handles arrays with mixed data types and returns the unique elements from both arrays.
Improve this sample solution and post your code through Disqus.
Previous:Write a JavaScript function to generate an array between two integers of 1 step length.
Next: Write a JavaScript function to create an array of arrays, ungrouping the elements in an array produced by zip.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.