JavaScript: Generate an array between two integers of 1 step length
JavaScript Array: Exercise-41 with Solution
Array Between Two Integers
Write a JavaScript function to generate an array between two integers of 1 step length.
Test Data :
console.log(rangeBetwee(4, 7));
[4, 5, 6, 7]
console.log(rangeBetwee(-4, 7));
[-4, -3, -2, -1, 0, 1, 2, 3, 4, 5, 6, 7]
Visual Presentation:
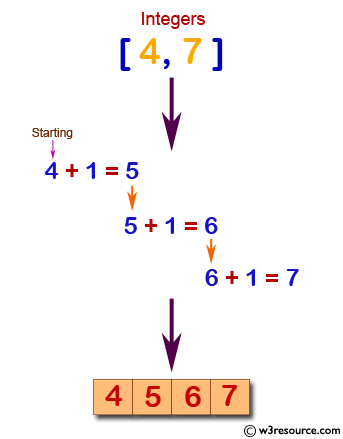
Sample Solution:
JavaScript Code:
// Function to generate an array of numbers between two values (inclusive)
function rangeBetween(start, end)
{
if (start > end) {
// If start is greater than end, create a new array and fill it in reverse order
const arr = Array.from({ length: start - end + 1 }, (_, index) => start - index);
return arr;
} else {
// If start is less than or equal to end, create a new array and fill it in ascending order
const arr = Array.from({ length: end - start + 1 }, (_, index) => start + index);
return arr;
}
}
// Output the result of generating an array between 4 and 7 (inclusive)
console.log(rangeBetween(4, 7));
// Output the result of generating an array between -4 and 7 (inclusive)
console.log(rangeBetween(-4, 7));
Output:
[4,5,6,7] [-4,-3,-2,-1,0,1,2,3,4,5,6,7]
Flowchart:
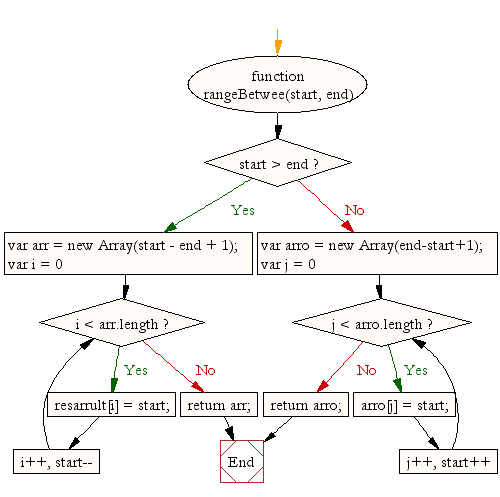
ES6 Version:
// Function to generate an array of numbers between two values (inclusive)
function rangeBetween(start, end) {
// Use conditional (ternary) operator to determine the direction of filling the array
const arr = start > end
? Array.from({ length: start - end + 1 }, (_, index) => start - index)
: Array.from({ length: end - start + 1 }, (_, index) => start + index);
// Return the generated array
return arr;
}
// Output the result of generating an array between 4 and 7 (inclusive)
console.log(rangeBetween(4, 7));
// Output the result of generating an array between -4 and 7 (inclusive)
console.log(rangeBetween(-4, 7));
Live Demo :
See the Pen JavaScript -Generate an array between two integers of 1 step length-array-ex- 41 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates an array containing all integers between two given numbers inclusively.
- Write a JavaScript function that handles cases where the starting integer is greater than the ending integer by swapping them.
- Write a JavaScript function that uses a for loop to generate all numbers between the two boundaries.
- Write a JavaScript function that validates the inputs to ensure they are valid integers for range generation.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to generate an array of specified length, filled with integer numbers, increase by one from starting position.
Next: Write a JavaScript function to find the unique elements from two arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.