JavaScript: Generate an array of specified length, the content of the array is integer numbers, increase by one from starting
JavaScript Array: Exercise-40 with Solution
Generate Integer Range Array
Write a JavaScript function to generate an array of integer numbers, increasing one from the starting position, of a specified length.
Test Data :
console.log(array_range(1, 4));
[1, 2, 3, 4]
console.log(array_range(-6, 4));
[-6, -5, -4, -3]
Visual Presentation:
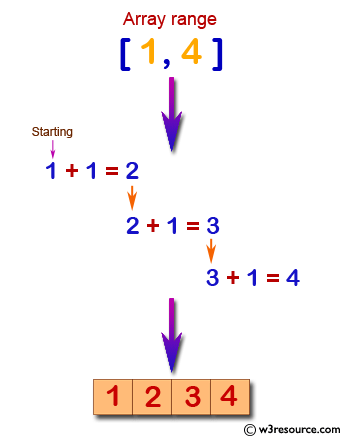
Sample Solution:
JavaScript Code:
// Function to generate an array of numbers in a specified range
function array_range(start, len)
{
// Create a new array with the specified length
var arr = new Array(len);
// Iterate through the array, filling it with values incremented from 'start'
for (var i = 0; i < len; i++, start++)
{
arr[i] = start;
}
// Return the generated array
return arr;
}
// Output the result of generating an array with a starting value of 1 and a length of 4
console.log(array_range(1, 4));
// Output the result of generating an array with a starting value of -6 and a length of 4
console.log(array_range(-6, 4));
Output:
[1,2,3,4] [-6,-5,-4,-3]
Flowchart:
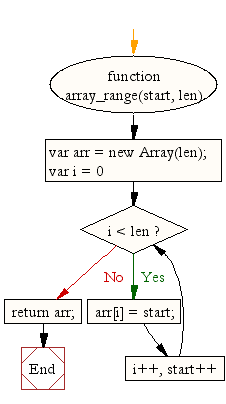
ES6 Version:
// Function to generate an array of numbers in a specified range
function array_range(start, len)
{
// Create a new array with the specified length using Array.from
const arr = Array.from({ length: len }, (_, index) => start + index);
// Return the generated array
return arr;
}
// Output the result of generating an array with a starting value of 1 and a length of 4
console.log(array_range(1, 4));
// Output the result of generating an array with a starting value of -6 and a length of 4
console.log(array_range(-6, 4));
Live Demo:
See the Pen JavaScript - Generate an array of specified length, the content of the array is integer numbers, increase by one from starting-array-ex- 40 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to filter false, null, 0 and blank values from an array.
Next: Write a JavaScript function to generate an array between two integers of 1 step length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics