JavaScript: Get the last element of an array
JavaScript Array: Exercise-4 with Solution
Last Elements of Array
Write a JavaScript function to get the last element of an array. Passing the parameter 'n' will return the last 'n' elements of the array.
Test Data :
console.log(last([7, 9, 0, -2]));
console.log(last([7, 9, 0, -2],3));
console.log(last([7, 9, 0, -2],6));
Visual Presentation:
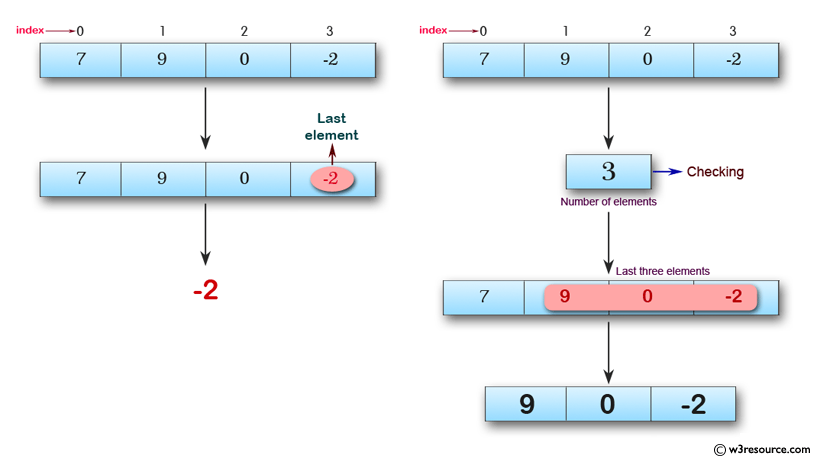
Sample Solution:
JavaScript Code:
// Function to get the last n elements of an array
var last = function(array, n) {
// Check if the input array is null, return undefined if true
if (array == null)
return void 0;
// Check if the value of n is null, return the last element of the array if true
if (n == null)
return array[array.length - 1];
// Use the slice method to get the last n elements of the array
// Math.max is used to ensure the starting index is not negative
return array.slice(Math.max(array.length - n, 0));
};
// Testing the function with various cases
console.log(last([7, 9, 0, -2]));
console.log(last([7, 9, 0, -2], 3));
console.log(last([7, 9, 0, -2], 6));
Output:
-2 [9,0,-2] [7,9,0,-2]
Flowchart:
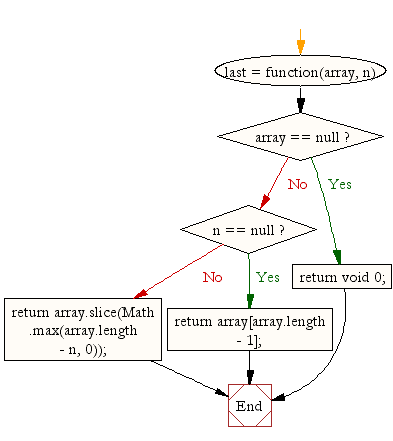
ES6 Version:
// Function to get the last n elements of an array
const last = (array, n) => {
// Check if the input array is null, return undefined if true
if (array == null)
return undefined;
// Check if the value of n is null, return the last element of the array if true
if (n == null)
return array[array.length - 1];
// Use the slice method to get the last n elements of the array
// Math.max is used to ensure the starting index is not negative
return array.slice(Math.max(array.length - n, 0));
};
// Testing the function with various cases
console.log(last([7, 9, 0, -2]));
console.log(last([7, 9, 0, -2], 3));
console.log(last([7, 9, 0, -2], 6));
Live Demo:
See the Pen JavaScript - Get the last element of an array- array-ex-4 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the last element of an array, or the last n elements if a number is provided.
- Write a JavaScript function that uses negative indexing to retrieve the last elements without reversing the array.
- Write a JavaScript function that handles edge cases such as an empty array or when n exceeds the array length.
- Write a JavaScript function that implements the retrieval of the last n elements recursively.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the first element of an array. Passing a parameter 'n' will return the first 'n' elements of the array.
Next: Write a simple JavaScript program to join all elements of the following array into a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.