JavaScript: Move an array element from one position to another
JavaScript Array: Exercise-38 with Solution
Move Array Element
Write a JavaScript function to move an array element from one position to another.
Test Data:
console.log(move([10, 20, 30, 40, 50], 0, 2));
[20, 30, 10, 40, 50]
console.log(move([10, 20, 30, 40, 50], -1, -2));
[10, 20, 30, 50, 40]
Visual Presentation:
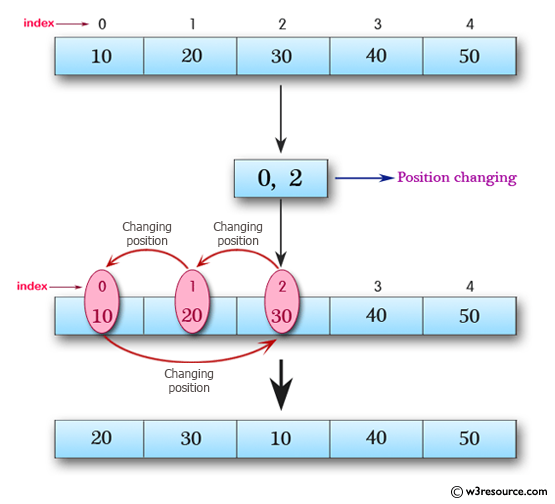
Sample Solution:
JavaScript Code:
// Function to move an element within an array from the 'old_index' to the 'new_index'
function move(arr, old_index, new_index) {
// Adjust negative indices to the equivalent positive indices
while (old_index < 0) {
old_index += arr.length;
}
while (new_index < 0) {
new_index += arr.length;
}
// If 'new_index' is beyond the array length, extend the array with undefined elements
if (new_index >= arr.length) {
var k = new_index - arr.length;
while ((k--) + 1) {
arr.push(undefined);
}
}
// Remove the element at 'old_index' and insert it at 'new_index'
arr.splice(new_index, 0, arr.splice(old_index, 1)[0]);
// Return the modified array
return arr;
}
// Output the result of moving the element at index 0 to index 2
console.log(move([10, 20, 30, 40, 50], 0, 2));
// Output the result of moving the last element to the second-to-last position
console.log(move([10, 20, 30, 40, 50], -1, -2));
Output:
[20,30,10,40,50] [10,20,30,50,40]
Flowchart:
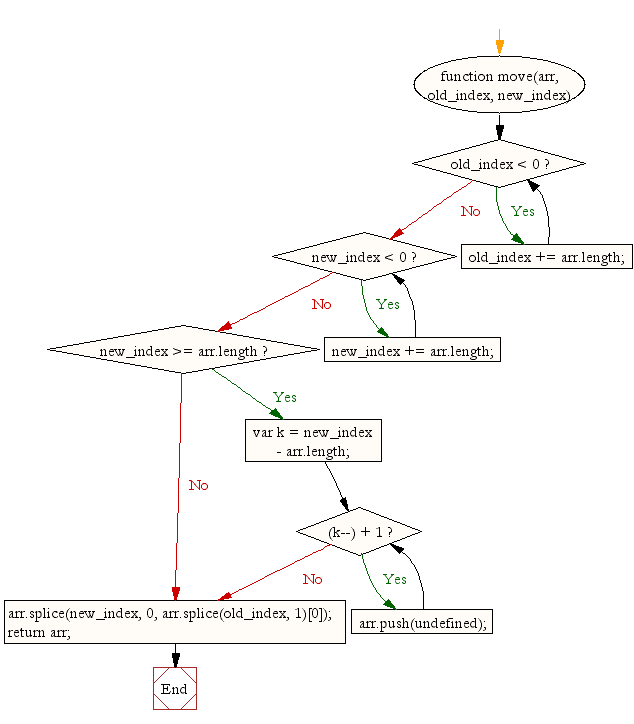
ES6 Version:
// Function to move an element within an array from the 'old_index' to the 'new_index'
function move(arr, old_index, new_index) {
// Adjust negative indices to the equivalent positive indices
while (old_index < 0) {
old_index += arr.length;
}
while (new_index < 0) {
new_index += arr.length;
}
// If 'new_index' is beyond the array length, extend the array with undefined elements
if (new_index >= arr.length) {
let k = new_index - arr.length;
// Use a loop to push undefined elements to the array
while ((k--) + 1) {
arr.push(undefined);
}
}
// Remove the element at 'old_index' and insert it at 'new_index'
arr.splice(new_index, 0, arr.splice(old_index, 1)[0]);
// Return the modified array
return arr;
}
// Output the result of moving the element at index 0 to index 2
console.log(move([10, 20, 30, 40, 50], 0, 2));
// Output the result of moving the last element to the second-to-last position
console.log(move([10, 20, 30, 40, 50], -1, -2));
Live Demo :
See the Pen JavaScript - Move an array element from one position to another-array-ex- 38 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that moves an element from one index to another within an array using splice().
- Write a JavaScript function that swaps two elements in an array by their positions without a temporary variable.
- Write a JavaScript function that shifts an element from one position to another and maintains the order of remaining elements.
- Write a JavaScript function that handles negative indices correctly when moving an element in an array.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to create a specified number of elements and pre-filled string value array.
Next: Write a JavaScript function to filter false, null, 0 and blank values from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.