JavaScript: Create a specified number of elements and pre-filled string value array
JavaScript Array: Exercise-37 with Solution
Pre-filled String Array
Write a JavaScript function to create a specified number of elements with a pre-filled string value array.
Test Data:
console.log(array_filled(3, 'default value'));
["default value", "default value", "default value"]
console.log(array_filled(4, 'password'));
["password", "password", "password", "password"]
Sample Solution:
JavaScript Code :
// Function to create a new array with 'n' elements, each initialized to the specified 'val'
function array_filled(n, val) {
// Use Array.apply to create an array-like object with 'n' undefined elements
// and then use map to fill each element with the specified 'val' converted to a string
return Array.apply(null, Array(n)).map(String.prototype.valueOf, val);
}
// Output the result of array_filled with 'n=3' and 'val='default value''
console.log(array_filled(3, 'default value'));
// Output the result of array_filled with 'n=4' and 'val='password''
console.log(array_filled(4, 'password'));
Output:
["default value","default value","default value"] ["password","password","password","password"]
Flowchart:
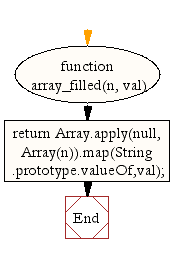
ES6 Version:
// Function to create a new array with 'n' elements, each initialized to the specified 'val'
const array_filled = (n, val) =>
// Use Array.from to create an array with 'n' undefined elements
Array.from({ length: n }, () => String(val));
// Output the result of array_filled with 'n=3' and 'val='default value''
console.log(array_filled(3, 'default value'));
// Output the result of array_filled with 'n=4' and 'val='password''
console.log(array_filled(4, 'password'));
Live Demo:
See the Pen JavaScript - Create a specified number of elements and pre-filled string value array-array-ex- 37 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to create a specified number of elements and pre-filled numeric value array.
Next: Write a JavaScript function to move an array element from one position to another.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics