JavaScript: Get a random item from an array
JavaScript Array: Exercise-35 with Solution
Random Array Item
Write a JavaScript function to get random items from an array.
Visual Presentation:
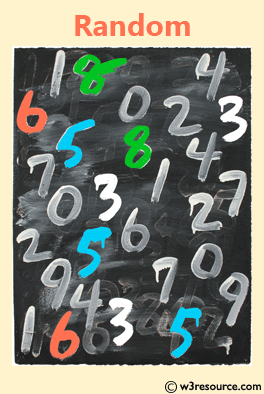
Sample Solution:
JavaScript Code:
// Function to return a random item from an array
function random_item(items) {
// Use Math.random() to generate a random number between 0 and 1,
// multiply it by the length of the array, and use Math.floor() to round down to the nearest integer
return items[Math.floor(Math.random() * items.length)];
}
// Declare and initialize an array of items
var items = [254, 45, 212, 365, 2543];
// Output the result of the random_item function with the array of items
console.log(random_item(items));
Output:
365
Flowchart:
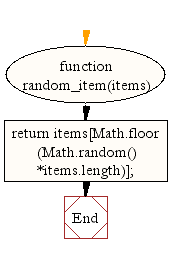
ES6 Version:
// Arrow function to return a random item from an array
const random_item = items => items[Math.floor(Math.random() * items.length)];
// Declare and initialize an array of items
const items = [254, 45, 212, 365, 2543];
// Output the result of the random_item function with the array of items
console.log(random_item(items));
Live Demo:
See the Pen JavaScript - Get a random item from an array - array-ex- 35 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns a random element from an array using Math.random() and floor.
- Write a JavaScript function that shuffles an array and returns its first element as a random item.
- Write a JavaScript function that generates a random index and retrieves the corresponding element from an array.
- Write a JavaScript function that handles empty arrays by returning a default value when picking a random item.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get nth largest element from an unsorted array.
Next: Write a JavaScript function to create a specified number of elements and pre-filled numeric value array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.