JavaScript: Get nth largest element from an unsorted array
JavaScript Array: Exercise-34 with Solution
Nth Largest Element
Write a JavaScript function to get the nth largest element from an unsorted array.
Test Data:
console.log(nthlargest([ 43, 56, 23, 89, 88, 90, 99, 652], 4));
89
Visual Presentation:
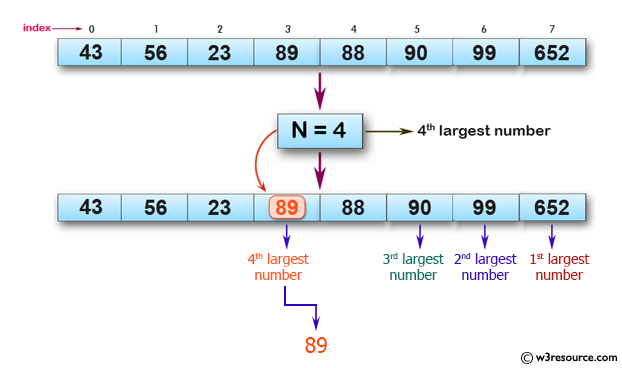
Sample Solution:
JavaScript Code:
// Function to find the nth largest element in an array
function nthlargest(arra, highest) {
// Initialize variables for array indices, temporary storage, array length, and flags
var x = 0,
y = 0,
z = 0,
temp = 0,
tnum = arra.length,
flag = false,
result = false;
// Iterate through the array while x is less than the array length
while (x < tnum) {
// Increment y to the next position
y = x + 1;
// Check if y is less than the array length
if (y < tnum) {
// Iterate through the array from y to the end
for (z = y; z < tnum; z++) {
// Check if the element at position x is less than the element at position z
if (arra[x] < arra[z]) {
// Swap the elements at positions x and z
temp = arra[z];
arra[z] = arra[x];
arra[x] = temp;
flag = true; // Set flag to true to indicate a swap
} else {
continue; // Continue to the next iteration if no swap is needed
}
}
}
// Check if a swap occurred
if (flag) {
flag = false; // Reset the flag
} else {
x++; // Move to the next position if no swap occurred
// Check if x is equal to the desired highest position
if (x === highest) {
result = true; // Set result flag to true if x matches the desired position
}
}
// Check if the result flag is set
if (result) {
break; // Break out of the loop if the result is found
}
}
// Return the nth largest element from the array
return arra[highest - 1];
}
// Output the result of the nthlargest function with a sample array and position
console.log(nthlargest([43, 56, 23, 89, 88, 90, 99, 652], 4));
Output:
89
Flowchart:
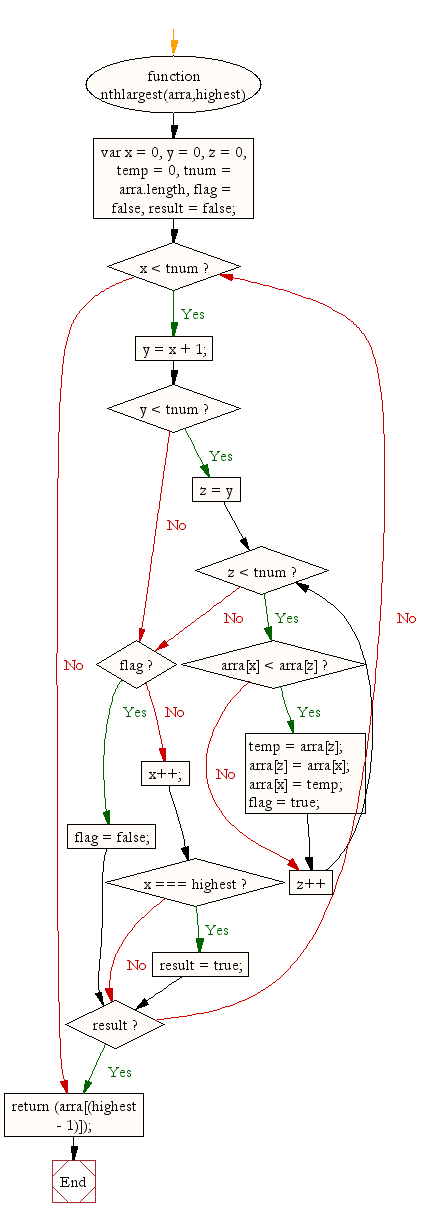
ES6 Version:
// Function to find the nth largest element in an array
function nthlargest(arra, highest) {
let x = 0,
y = 0,
z = 0,
temp = 0,
tnum = arra.length,
flag = false,
result = false;
// Iterate through the array
while (x < tnum) {
y = x + 1;
// Check if y is less than the array length
if (y < tnum) {
// Iterate through the array from y to the end
for (z = y; z < tnum; z++) {
// Check if the current element is greater than the element at position x
if (arra[x] < arra[z]) {
// Swap the elements
temp = arra[z];
arra[z] = arra[x];
arra[x] = temp;
flag = true;
} else {
continue;
}
}
}
// Check if a swap occurred
if (flag) {
flag = false;
} else {
// Move to the next position
x++;
// Check if x is equal to the desired highest position
if (x === highest) {
result = true;
}
}
// Check if the result is found
if (result) {
break;
}
}
return arra[highest - 1];
}
// Output the result of the nthlargest function with a sample array and position
console.log(nthlargest([43, 56, 23, 89, 88, 90, 99, 652], 4));
Live Demo:
See the Pen JavaScript - Get nth largest element from an unsorted array - array-ex- 34 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the nth largest element in an unsorted array by sorting it first.
- Write a JavaScript function that uses a partial selection sort to determine the nth largest element efficiently.
- Write a JavaScript function that implements a max heap to extract the nth largest element without full sorting.
- Write a JavaScript function that handles duplicates by returning the nth largest unique element from the array.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript script to empty an array keeping the original.
Next: Write a JavaScript function to get a random item from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.