JavaScript: Merge two arrays and removes all duplicates elements
JavaScript Array: Exercise-30 with Solution
Merge Arrays Without Duplicates
Write a JavaScript function that merges two arrays and removes all duplicate elements.
Test data:
var array1 = [1, 2, 3];
var array2 = [2, 30, 1];
console.log(merge_array(array1, array2));
[3, 2, 30, 1]
Visual Presentation:
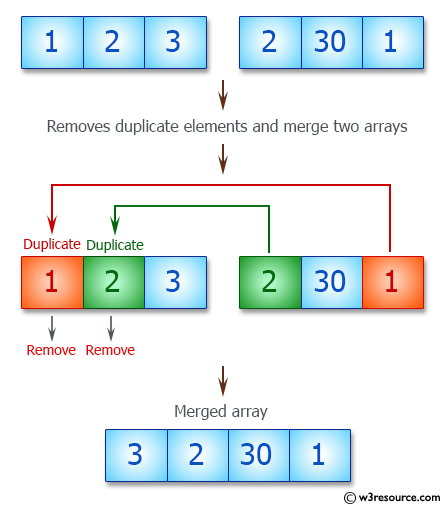
Sample Solution:
JavaScript Code:
// Function to merge two arrays, removing duplicates and maintaining the order
function merge_array(array1, array2) {
// Initialize an empty array to store the result
var result_array = [];
// Concatenate both arrays to form a new array
var arr = array1.concat(array2);
// Get the length of the new array
var len = arr.length;
// Initialize an empty object to keep track of unique items
var assoc = {};
// Iterate through the array in reverse order
while (len--) {
// Get the current item from the array
var item = arr[len];
// Check if the item is not already in the result array
if (!assoc[item]) {
// Add the item to the beginning of the result array
result_array.unshift(item);
// Mark the item as seen in the associative object
assoc[item] = true;
}
}
// Return the result array with unique items
return result_array;
}
// Example arrays for testing
var array1 = [1, 2, 3];
var array2 = [2, 30, 1];
// Output the result of merging the arrays
console.log(merge_array(array1, array2));
Output:
[3,2,30,1]
Flowchart:
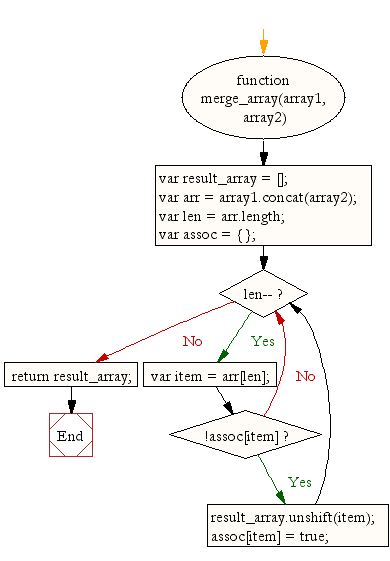
ES6 Version:
// Function to merge two arrays, removing duplicates and maintaining the order
const mergeArray = (array1, array2) => {
// Initialize an empty array to store the result
const resultArray = [];
// Concatenate both arrays to form a new array
const arr = array1.concat(array2);
// Get the length of the new array
let len = arr.length;
// Initialize an empty object to keep track of unique items
const assoc = {};
// Iterate through the array in reverse order
while (len--) {
// Get the current item from the array
const item = arr[len];
// Check if the item is not already in the result array
if (!assoc[item]) {
// Add the item to the beginning of the result array
resultArray.unshift(item);
// Mark the item as seen in the associative object
assoc[item] = true;
}
}
// Return the result array with unique items
return resultArray;
};
// Example arrays for testing
const array1 = [1, 2, 3];
const array2 = [2, 30, 1];
// Output the result of merging the arrays
console.log(mergeArray(array1, array2));
Live Demo:
See the Pen JavaScript - Merge two arrays and removes all duplicates elements - array-ex- 30 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that merges two arrays and removes duplicates using the Set data structure.
- Write a JavaScript function that combines two arrays and filters out duplicates using the filter() method.
- Write a JavaScript function that manually iterates through both arrays and adds only unique elements to a new array.
- Write a JavaScript function that handles merging arrays with mixed data types and returns a duplicate-free array.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to fill an array with values (numeric, string with one character) on supplied bounds.
Next: Write a JavaScript function to remove a specific element from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.