JavaScript: Find the longest common starting substring in a set of strings
JavaScript Array: Exercise-28 with Solution
Longest Common Starting Substring
Write a JavaScript function to find the longest common starting substring in a set of strings.
Sample array : console.log(longest_common_starting_substring(['go', 'google']));
Expected result : "go"
Visual Presentation:
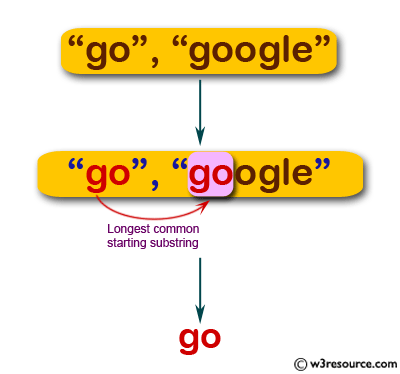
Sample Solution:
JavaScript Code:
// Function to find the longest common starting substring in an array of strings
function longest_common_starting_substring(arr1) {
// Create a sorted copy of the input array
var arr = arr1.concat().sort(),
// Get the first and last strings after sorting
a1 = arr[0],
a2 = arr[arr.length - 1],
// Get the length of the first string
L = a1.length,
// Initialize an index variable
i = 0;
// Iterate through the characters of the first string until a mismatch is found
while (i < L && a1.charAt(i) === a2.charAt(i)) {
i++;
}
// Return the longest common starting substring using substring(0, i)
return a1.substring(0, i);
}
// Output the result of the longest_common_starting_substring function with sample arrays
console.log(longest_common_starting_substring(['go', 'google'])); // Output: 'go'
console.log(longest_common_starting_substring(['SQLInjection', 'SQLTutorial'])); // Output: 'SQL'
console.log(longest_common_starting_substring(['abcd', '1234']));
Output:
go SQL
Flowchart:
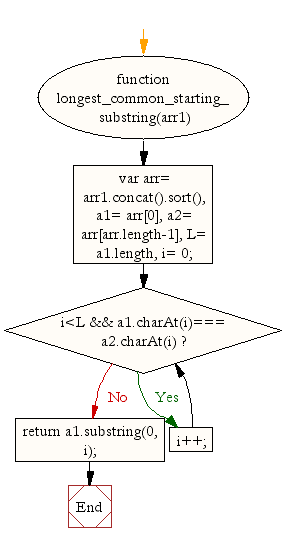
ES6 Version:
// Function to find the longest common starting substring in an array of strings
const longest_common_starting_substring = (arr1) => {
// Create a sorted copy of the input array
const arr = [...arr1].sort();
// Get the first and last strings after sorting
const a1 = arr[0];
const a2 = arr[arr.length - 1];
// Get the length of the first string
const L = a1.length;
// Initialize an index variable
let i = 0;
// Iterate through the characters of the first string until a mismatch is found
while (i < L && a1.charAt(i) === a2.charAt(i)) {
i++;
}
// Return the longest common starting substring using substring(0, i)
return a1.substring(0, i);
};
// Output the result of the longest_common_starting_substring function with sample arrays
console.log(longest_common_starting_substring(['go', 'google'])); // Output: 'go'
console.log(longest_common_starting_substring(['SQLInjection', 'SQLTutorial'])); // Output: 'SQL'
console.log(longest_common_starting_substring(['abcd', '1234']));
Live Demo:
See the Pen JavaScript - Find the longest common starting substring in a set of strings - array-ex- 28 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the longest common prefix among an array of strings using horizontal scanning.
- Write a JavaScript function that uses vertical scanning to determine the longest common starting substring.
- Write a JavaScript function that handles edge cases such as empty arrays and returns an empty string if no common prefix exists.
- Write a JavaScript function that iterates character by character to find the common starting substring of a set of strings.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to retrieve the value of a given property from all elements in an array.
Next: Write a JavaScript function to fill an array with values (numeric, string with one character) on supplied bounds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics